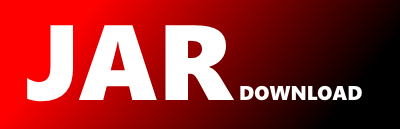
com.github.krukow.clj_ds.Transients Maven / Gradle / Ivy
package com.github.krukow.clj_ds;
import com.github.krukow.clj_lang.PersistentArrayMap;
import com.github.krukow.clj_lang.PersistentHashMap;
import com.github.krukow.clj_lang.PersistentHashSet;
import com.github.krukow.clj_lang.PersistentVector;
public final class Transients {
// Factory Methods
public static final TransientVector transientVector() {
return PersistentVector.emptyVector().asTransient();
}
public static final TransientSet transientHashSet() {
return PersistentHashSet.emptySet().asTransient();
}
@SuppressWarnings("unchecked")
public static final TransientMap transientHashMap() {
return PersistentHashMap.emptyMap().asTransient();
}
@SuppressWarnings("unchecked")
public static final TransientMap transientArrayMap() {
return PersistentArrayMap.EMPTY.asTransient();
}
// Utilities
public static TransientVector plusAll(TransientVector vec, Iterable extends E> others) {
TransientVector tv = vec;
for (E other : others) {
tv = tv.plus(other);
}
return tv;
}
public static TransientVector plusAll(TransientVector vec, E... others) {
TransientVector tv = vec;
for (E other : others) {
tv = tv.plus(other);
}
return tv;
}
public static TransientSet plusAll(TransientSet set, Iterable extends E> others) {
TransientSet tv = set;
for (E other : others) {
tv = tv.plus(other);
}
return tv;
}
public static TransientSet plusAll(TransientSet set, E... others) {
TransientSet tv = set;
for (E other : others) {
tv = tv.plus(other);
}
return tv;
}
public static TransientSet minusAll(TransientSet set, Iterable extends E> others) {
TransientSet tv = set;
for (E other : others) {
tv = tv.minus(other);
}
return tv;
}
private Transients() {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy