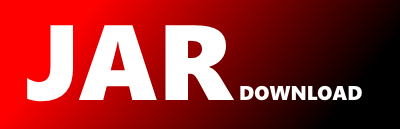
com.github.krukow.clj_lang.APersistentVector Maven / Gradle / Ivy
/**
* Copyright (c) Rich Hickey. All rights reserved.
* The use and distribution terms for this software are covered by the
* Eclipse Public License 1.0 (http://opensource.org/licenses/eclipse-1.0.php)
* which can be found in the file epl-v10.html at the root of this distribution.
* By using this software in any fashion, you are agreeing to be bound by
* the terms of this license.
* You must not remove this notice, or any other, from this software.
**/
/* rich Dec 18, 2007 */
package com.github.krukow.clj_lang;
import java.io.Serializable;
import java.util.Collection;
import java.util.Iterator;
import java.util.List;
import java.util.ListIterator;
import java.util.RandomAccess;
public abstract class APersistentVector extends AFn implements IPersistentVector, Iterable,
List,
RandomAccess, Comparable,
Serializable, IHashEq {
int _hash = -1;
int _hasheq = -1;
public String toString(){
return RT.printString(this);
}
public ISeq seq(){
if(count() > 0)
return new Seq(this, 0);
return null;
}
public ISeq rseq(){
if(count() > 0)
return new RSeq(this, count() - 1);
return null;
}
static boolean doEquals(IPersistentVector v, Object obj){
if(v == obj) return true;
if(obj instanceof List || obj instanceof IPersistentVector)
{
Collection ma = (Collection) obj;
if(ma.size() != v.count() || ma.hashCode() != v.hashCode())
return false;
for(Iterator i1 = ((List) v).iterator(), i2 = ma.iterator();
i1.hasNext();)
{
if(!Util.equals(i1.next(), i2.next()))
return false;
}
return true;
}
// if(obj instanceof IPersistentVector)
// {
// IPersistentVector ma = (IPersistentVector) obj;
// if(ma.count() != v.count() || ma.hashCode() != v.hashCode())
// return false;
// for(int i = 0; i < v.count(); i++)
// {
// if(!Util.equal(v.nth(i), ma.nth(i)))
// return false;
// }
// }
else
{
if(!(obj instanceof Sequential))
return false;
ISeq ms = RT.seq(obj);
for(int i = 0; i < v.count(); i++, ms = ms.next())
{
if(ms == null || !Util.equals(v.nth(i), ms.first()))
return false;
}
if(ms != null)
return false;
}
return true;
}
static boolean doEquiv(IPersistentVector v, Object obj){
if(obj instanceof List || obj instanceof IPersistentVector)
{
Collection ma = (Collection) obj;
if(ma.size() != v.count())
return false;
for(Iterator i1 = ((List) v).iterator(), i2 = ma.iterator();
i1.hasNext();)
{
if(!Util.equiv(i1.next(), i2.next()))
return false;
}
return true;
}
// if(obj instanceof IPersistentVector)
// {
// IPersistentVector ma = (IPersistentVector) obj;
// if(ma.count() != v.count() || ma.hashCode() != v.hashCode())
// return false;
// for(int i = 0; i < v.count(); i++)
// {
// if(!Util.equal(v.nth(i), ma.nth(i)))
// return false;
// }
// }
else
{
if(!(obj instanceof Sequential))
return false;
ISeq ms = RT.seq(obj);
for(int i = 0; i < v.count(); i++, ms = ms.next())
{
if(ms == null || !Util.equiv(v.nth(i), ms.first()))
return false;
}
if(ms != null)
return false;
}
return true;
}
public boolean equals(Object obj){
return doEquals(this, obj);
}
public boolean equiv(Object obj){
return doEquiv(this, obj);
}
public int hashCode(){
if(_hash == -1)
{
int hash = 1;
Iterator i = iterator();
while(i.hasNext())
{
Object obj = i.next();
hash = 31 * hash + (obj == null ? 0 : obj.hashCode());
}
// int hash = 0;
// for(int i = 0; i < count(); i++)
// {
// hash = Util.hashCombine(hash, Util.hash(nth(i)));
// }
this._hash = hash;
}
return _hash;
}
public int hasheq(){
if(_hasheq == -1) {
int hash = 1;
Iterator i = iterator();
while(i.hasNext())
{
Object obj = i.next();
hash = 31 * hash + Util.hasheq(obj);
}
_hasheq = hash;
}
return _hasheq;
}
public T get(int index){
return nth(index);
}
public T nth(int i, T notFound){
if(i >= 0 && i < count())
return nth(i);
return notFound;
}
public T remove(int i){
throw new UnsupportedOperationException();
}
public int indexOf(Object o){
for(int i = 0; i < count(); i++)
if(Util.equiv(nth(i), o))
return i;
return -1;
}
public int lastIndexOf(Object o){
for(int i = count() - 1; i >= 0; i--)
if(Util.equiv(nth(i), o))
return i;
return -1;
}
public ListIterator listIterator(){
return listIterator(0);
}
public ListIterator listIterator(final int index){
return new ListIterator(){
int nexti = index;
public boolean hasNext(){
return nexti < count();
}
public T next(){
return nth(nexti++);
}
public boolean hasPrevious(){
return nexti > 0;
}
public T previous(){
return nth(--nexti);
}
public int nextIndex(){
return nexti;
}
public int previousIndex(){
return nexti - 1;
}
public void remove(){
throw new UnsupportedOperationException();
}
public void set(Object o){
throw new UnsupportedOperationException();
}
public void add(Object o){
throw new UnsupportedOperationException();
}
};
}
@SuppressWarnings("unchecked")
public List subList(int fromIndex, int toIndex){
return (List) RT.subvec(this, fromIndex, toIndex);
}
public T set(int i, T o){
throw new UnsupportedOperationException();
}
public void add(int i, T o){
throw new UnsupportedOperationException();
}
public boolean addAll(int i, Collection c){
throw new UnsupportedOperationException();
}
public Object invoke(Object arg1) {
if(Util.isInteger(arg1))
return nth(((Number) arg1).intValue());
throw new IllegalArgumentException("Key must be integer");
}
public Iterator iterator(){
//todo - something more efficient
return new Iterator(){
int i = 0;
public boolean hasNext(){
return i < count();
}
public T next(){
return nth(i++);
}
public void remove(){
throw new UnsupportedOperationException();
}
};
}
public T peek(){
if(count() > 0)
return nth(count() - 1);
return null;
}
public boolean containsKey(Object key){
if(!(Util.isInteger(key)))
return false;
int i = ((Number) key).intValue();
return i >= 0 && i < count();
}
public IMapEntry
© 2015 - 2025 Weber Informatics LLC | Privacy Policy