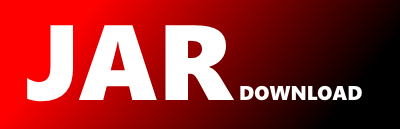
com.github.krukow.clj_lang.PersistentHashSet Maven / Gradle / Ivy
/**
* Copyright (c) Rich Hickey. All rights reserved.
* The use and distribution terms for this software are covered by the
* Eclipse Public License 1.0 (http://opensource.org/licenses/eclipse-1.0.php)
* which can be found in the file epl-v10.html at the root of this distribution.
* By using this software in any fashion, you are agreeing to be bound by
* the terms of this license.
* You must not remove this notice, or any other, from this software.
**/
/* rich Mar 3, 2008 */
package com.github.krukow.clj_lang;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import com.github.krukow.clj_ds.PersistentSet;
import com.github.krukow.clj_ds.TransientSet;
public class PersistentHashSet extends APersistentSet implements IObj, IEditableCollection, PersistentSet {
static public final PersistentHashSet EMPTY = new PersistentHashSet(null, PersistentHashMap.EMPTY);
@SuppressWarnings("unchecked")
static public final PersistentHashSet emptySet() {
return EMPTY;
}
final IPersistentMap _meta;
public static PersistentHashSet create(T... init){
PersistentHashSet ret = EMPTY;
for(int i = 0; i < init.length; i++)
{
ret = (PersistentHashSet) ret.cons(init[i]);
}
return ret;
}
public static PersistentHashSet create(Iterable extends T> init){
PersistentHashSet ret = EMPTY;
for(T key : init)
{
ret = (PersistentHashSet) ret.cons(key);
}
return ret;
}
static public PersistentHashSet create(ISeq extends T> items){
PersistentHashSet ret = EMPTY;
for(; items != null; items = items.next())
{
ret = (PersistentHashSet) ret.cons(items.first());
}
return ret;
}
public static PersistentHashSet createWithCheck(T ... init){
PersistentHashSet ret = EMPTY;
for(int i = 0; i < init.length; i++)
{
ret = (PersistentHashSet) ret.cons(init[i]);
if(ret.count() != i + 1)
throw new IllegalArgumentException("Duplicate key: " + init[i]);
}
return ret;
}
public static PersistentHashSet createWithCheck(List extends T> init){
PersistentHashSet ret = EMPTY;
int i=0;
for(T key : init)
{
ret = (PersistentHashSet) ret.cons(key);
if(ret.count() != i + 1)
throw new IllegalArgumentException("Duplicate key: " + key);
++i;
}
return ret;
}
static public PersistentHashSet createWithCheck(ISeq extends T> items){
PersistentHashSet ret = EMPTY;
for(int i=0; items != null; items = items.next(), ++i)
{
ret = (PersistentHashSet) ret.cons(items.first());
if(ret.count() != i + 1)
throw new IllegalArgumentException("Duplicate key: " + items.first());
}
return ret;
}
PersistentHashSet(IPersistentMap meta, IPersistentMap impl){
super(impl);
this._meta = meta;
}
public Iterator iterator(){
return new Iterator() {
final Iterator iterator = impl.iterator();
public boolean hasNext() {
return iterator.hasNext();
}
public T next() {
Map.Entry n = iterator.next();
return (T) n.getKey();
}
@Override
public void remove() {
throw new UnsupportedOperationException();
}
};
}
public PersistentHashSet disjoin(T key) {
if(contains(key))
return new PersistentHashSet(meta(),impl.without(key));
return this;
}
public PersistentHashSet cons(T o){
if(contains(o))
return this;
return new PersistentHashSet(meta(),impl.assoc(o,o));
}
public IPersistentSet empty(){
return EMPTY.withMeta(meta());
}
public PersistentHashSet withMeta(IPersistentMap meta){
return new PersistentHashSet(meta, impl);
}
public TransientHashSet asTransient() {
return new TransientHashSet(((PersistentHashMap) impl).asTransient());
}
public IPersistentMap meta(){
return _meta;
}
static final class TransientHashSet extends ATransientSet implements TransientSet {
TransientHashSet(ITransientMap impl) {
super(impl);
}
public PersistentHashSet persistent() {
return new PersistentHashSet(null, impl.persistentMap());
}
@Override
public PersistentSet persist() {
return persistent();
}
@Override
public TransientSet plus(T val) {
return (TransientSet) conj(val);
}
@Override
public TransientSet minus(T val) {
return (TransientSet) disjoin(val);
}
}
@Override
public PersistentSet zero() {
return (PersistentSet) empty();
}
@Override
public PersistentSet plus(T val) {
return cons(val);
}
@Override
public PersistentSet minus(T val) {
return disjoin(val);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy