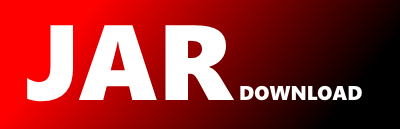
com.github.krukow.clj_lang.PersistentTreeSet Maven / Gradle / Ivy
/**
* Copyright (c) Rich Hickey. All rights reserved.
* The use and distribution terms for this software are covered by the
* Eclipse Public License 1.0 (http://opensource.org/licenses/eclipse-1.0.php)
* which can be found in the file epl-v10.html at the root of this distribution.
* By using this software in any fashion, you are agreeing to be bound by
* the terms of this license.
* You must not remove this notice, or any other, from this software.
**/
/* rich Mar 3, 2008 */
package com.github.krukow.clj_lang;
import java.util.Comparator;
import com.github.krukow.clj_ds.PersistentSortedSet;
import com.github.krukow.clj_ds.TransientCollection;
public class PersistentTreeSet extends APersistentSet implements IObj, Reversible, Sorted, PersistentSortedSet{
static public final PersistentTreeSet EMPTY = new PersistentTreeSet(null, PersistentTreeMap.EMPTY);
final IPersistentMap _meta;
static public PersistentTreeSet create(ISeq extends T> items){
PersistentTreeSet ret = EMPTY;
for(; items != null; items = items.next())
{
ret = (PersistentTreeSet) ret.cons(items.first());
}
return ret;
}
static public PersistentTreeSet create(Comparator comp, ISeq extends T> items){
PersistentTreeSet ret = new PersistentTreeSet(null, new PersistentTreeMap(null, comp));
for(; items != null; items = items.next())
{
ret = (PersistentTreeSet) ret.cons(items.first());
}
return ret;
}
PersistentTreeSet(IPersistentMap meta, IPersistentMap impl){
super(impl);
this._meta = meta;
}
public PersistentTreeSet disjoin(T key) {
if(contains(key))
return new PersistentTreeSet(meta(),impl.without(key));
return this;
}
public PersistentTreeSet cons(T o){
if(contains(o))
return this;
return new PersistentTreeSet(meta(),impl.assoc(o,o));
}
public PersistentTreeSet empty(){
return new PersistentTreeSet(meta(),(PersistentTreeMap)impl.empty());
}
public ISeq rseq() {
return APersistentMap.KeySeq.create(((Reversible) impl).rseq());
}
public PersistentTreeSet withMeta(IPersistentMap meta){
return new PersistentTreeSet(meta, impl);
}
public Comparator comparator(){
return ((Sorted)impl).comparator();
}
public Object entryKey(Object entry){
return entry;
}
public ISeq seq(boolean ascending){
PersistentTreeMap m = (PersistentTreeMap) impl;
return RT.keys(m.seq(ascending));
}
public ISeq seqFrom(T key, boolean ascending){
PersistentTreeMap m = (PersistentTreeMap) impl;
return RT.keys(m.seqFrom(key,ascending));
}
public IPersistentMap meta(){
return _meta;
}
@Override
public PersistentSortedSet zero() {
return empty();
}
@Override
public PersistentSortedSet plus(T o) {
return cons(o);
}
@Override
public PersistentSortedSet minus(T key) {
return disjoin(key);
}
@Override
public TransientCollection asTransient() {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy