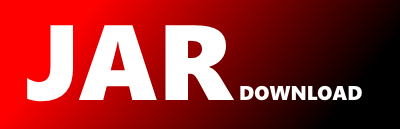
com.ksyun.api.sdk.client.DefaultKscClient Maven / Gradle / Ivy
package com.ksyun.api.sdk.client;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.net.SocketTimeoutException;
import java.security.InvalidKeyException;
import java.util.List;
import com.ksc.KscWebServiceRequest;
import com.ksc.auth.BasicAWSCredentials;
import com.ksc.auth.credentials.AWSCredentials;
import com.ksc.util.ReflectUtils;
import com.ksc.util.StringUtils;
import com.ksyun.api.sdk.auth.Credential;
import com.ksyun.api.sdk.auth.ISigner;
import com.ksyun.api.sdk.exceptions.ClientException;
import com.ksyun.api.sdk.exceptions.ServerException;
import com.ksyun.api.sdk.http.FormatType;
import com.ksyun.api.sdk.http.UnmarshallerContext;
import com.ksyun.api.sdk.regions.Endpoint;
import com.ksyun.api.sdk.regions.ProductDomain;
import com.ksyun.api.sdk.reponse.HttpResponse;
import com.ksyun.api.sdk.reponse.KscError;
import com.ksyun.api.sdk.reponse.KscResponse;
import com.ksyun.api.sdk.request.HttpRequest;
import com.ksyun.api.sdk.request.KscRequest;
import com.ksyun.api.sdk.utils.Reader;
import com.ksyun.api.sdk.utils.ReaderFactory;
public class DefaultKscClient implements IKscClient{
private int maxRetryNumber = 3;
private boolean autoRetry = true;
private IClientProfile clientProfile = null;
private boolean urlTestFlag = false;
private static String DEFAULT_ENDPOINT = "http://%s.%s.api.ksyun.com";
public DefaultKscClient() {
this.clientProfile = DefaultKscProfile.getProfile();
}
public DefaultKscClient(String region,String ak,String sk) {
this.clientProfile = DefaultKscProfile.getProfile(region,ak,sk);
}
public DefaultKscClient(IClientProfile profile) {
this.clientProfile = profile;
}
@Override
public HttpResponse doAction(KscRequest request)
throws ClientException, ServerException {
return this.doAction(request, autoRetry, maxRetryNumber, this.clientProfile);
}
@Override
public HttpResponse doAction(KscRequest request,
boolean autoRetry, int maxRetryCounts) throws ClientException, ServerException {
return this.doAction(request, autoRetry, maxRetryCounts, this.clientProfile);
}
@Override
public HttpResponse doAction(KscRequest request, IClientProfile profile)
throws ClientException, ServerException {
return this.doAction(request, this.autoRetry, this.maxRetryNumber, profile);
}
@Override
public HttpResponse doAction(KscRequest request, String regionId, Credential credential)
throws ClientException, ServerException {
boolean retry = this.autoRetry;
int retryNumber = this.maxRetryNumber;
ISigner signer = null;
FormatType format = null;
List endpoints = null;
if (null == request.getRegionId()) {
request.setRegionId(regionId);
}
if (null != this.clientProfile) {
signer = clientProfile.getSigner();
format = clientProfile.getFormat();
try {
endpoints = clientProfile.getEndpoints(request.getProduct(), request.getRegionId(),
request.getLocationProduct(),
request.getEndpointType());
} catch (Throwable e) {
endpoints = clientProfile.getEndpoints(request.getRegionId(), request.getProduct());
}
}
return this.doAction(request, retry, retryNumber, request.getRegionId(), credential, signer, format, endpoints);
}
@Override
public T getKscResponse(KscRequest request)
throws ServerException, ClientException{
HttpResponse baseResponse = this.doAction(request);
return parseKscResponse(request.getResponseClass(), baseResponse);
}
@Override
public T getKscResponse(KscRequest request,
boolean autoRetry, int maxRetryCounts) throws ServerException, ClientException{
HttpResponse baseResponse = this.doAction(request, autoRetry, maxRetryCounts);
return parseKscResponse(request.getResponseClass(), baseResponse);
}
@Override
public T getKscResponse(KscRequest request,IClientProfile profile)
throws ServerException, ClientException{
HttpResponse baseResponse = this.doAction(request, profile);
return parseKscResponse(request.getResponseClass(), baseResponse);
}
@Override
public T getKscResponse(KscRequest request, String regionId, Credential credential)
throws ServerException, ClientException {
HttpResponse baseResponse = this.doAction(request, regionId, credential);
return parseKscResponse(request.getResponseClass(), baseResponse);
}
@Override
public HttpResponse doAction(KscRequest request, boolean autoRetry,
int maxRetryCounts, IClientProfile profile) throws ClientException, ServerException {
if (null == profile){
throw new ClientException("SDK.InvalidProfile", "No active profile found.");
}
boolean retry = autoRetry;
int retryNumber = maxRetryCounts;
String region = profile.getRegionId();
if (null == request.getRegionId()) {
request.setRegionId(region);
}
Credential credential = profile.getCredential();
ISigner signer = profile.getSigner();
FormatType format = profile.getFormat();
List endpoints;
try {
endpoints = clientProfile.getEndpoints(request.getProduct(), request.getRegionId(),
request.getLocationProduct(),
request.getEndpointType());
} catch (Throwable e) {
endpoints = clientProfile.getEndpoints(request.getRegionId(), request.getProduct());
}
return this.doAction(request, retry, retryNumber, request.getRegionId(), credential, signer, format, endpoints);
}
private T parseKscResponse(Class clasz, HttpResponse baseResponse)
throws ServerException, ClientException {
FormatType format = baseResponse.getContentType();
if (baseResponse.isSuccess()) {
return readResponse(clasz, baseResponse, format);
} else {
KscError error = readError(baseResponse, format);
if (500 <= baseResponse.getStatus()){
throw new ServerException(error.getErrorCode(), error.getErrorMessage(), error.getRequestId());
}
else{
throw new ClientException(error.getErrorCode(), error.getErrorMessage(), error.getRequestId());
}
}
}
@Override
public HttpResponse doAction(KscRequest request,
boolean autoRetry, int maxRetryNumber,
String regionId, Credential credential,
ISigner signer, FormatType format,
List endpoints) throws ClientException, ServerException {
try {
FormatType requestFormatType = request.getAcceptFormat();
if (null != requestFormatType){
format = requestFormatType;
}
ProductDomain domain = Endpoint.findProductDomain(endpoints);
// ProductDomain domain = Endpoint.findProductDomain(regionId, request.getProduct(), endpoints);
if (null == domain){
throw new ClientException("SDK.InvalidRegionId", "Can not find endpoint to access.");
}
HttpRequest httpRequest = request.signRequest(signer, credential, format, domain);
if (this.urlTestFlag) {
throw new ClientException("URLTestFlagIsSet", httpRequest.getUrl());
}
int retryTimes = 1;
HttpResponse response = HttpResponse.getResponse(httpRequest);
while (500 <= response.getStatus() && autoRetry && retryTimes < maxRetryNumber) {
httpRequest = request.signRequest(signer, credential, format, domain);
response = HttpResponse.getResponse(httpRequest);
retryTimes ++;
}
return response;
} catch (InvalidKeyException exp) {
throw new ClientException("SDK.InvalidAccessSecret","Speicified access secret is not valid.");
}catch (SocketTimeoutException exp){
throw new ClientException("SDK.ServerUnreachable","SocketTimeoutException has occurred on a socket read or accept.");
}catch (IOException exp) {
throw new ClientException("SDK.ServerUnreachable", "Server unreachable: " + exp.toString());
}
}
@SuppressWarnings({ "rawtypes", "unchecked" })
@Override
public T getKscResponse(KscWebServiceRequest request) throws ClientException {
if(clientProfile.getCredential() == null
|| StringUtils.isNullOrEmpty(clientProfile.getCredential().getAccessKeyId())
|| StringUtils.isNullOrEmpty(clientProfile.getCredential().getAccessSecret()))
throw new ClientException("AK&SK cannot be null!");
//redirect to ksc client
AWSCredentials credentials = new BasicAWSCredentials(clientProfile.getCredential().getAccessKeyId()
, clientProfile.getCredential().getAccessSecret());
Constructor constructor;
Object client;
T t = null;
try {
//KSCKECClient
constructor = ReflectUtils.findConstructor(request.getReflectConstructureName());
client = constructor.newInstance(credentials);
//set endpoint
invokeEndpoint(request.getProduct(), clientProfile.getRegionId(), client, request);
t = (T) ReflectUtils.findMethod(request.getReflectMethodName()).invoke(client, request);
} catch (InstantiationException | IllegalAccessException | InvocationTargetException e) {
throw new RuntimeException("KSC SDK InstantiationException",e);
}
return t;
}
private static void invokeEndpoint(String product,String region,Object client,KscWebServiceRequest request)
throws IllegalAccessException, IllegalArgumentException, InvocationTargetException {
String endpoint = String.format(DEFAULT_ENDPOINT, product,region);
ReflectUtils.findMethod(request.getReflectEndpointMethodName()).invoke(client, endpoint);
}
private T readResponse(Class clasz, HttpResponse httpResponse, FormatType format) throws ClientException {
Reader reader = ReaderFactory.createInstance(format);
UnmarshallerContext context = new UnmarshallerContext();
T response = null;
String stringContent = getResponseContent(httpResponse);
try {
response = clasz.newInstance();
} catch (Exception e) {
throw new ClientException("SDK.InvalidResponseClass", "Unable to allocate "+ clasz.getName() + " class");
}
String responseEndpoint= clasz.getName().substring(clasz.getName().lastIndexOf(".") + 1);
context.setResponseMap(reader.read(stringContent,responseEndpoint));
context.setHttpResponse(httpResponse);
response.getInstance(context);
return response;
}
private String getResponseContent(HttpResponse httpResponse) throws ClientException {
String stringContent = null;
try {
if(null == httpResponse.getEncoding()){
stringContent = new String(httpResponse.getContent());
} else {
stringContent = new String(httpResponse.getContent(), httpResponse.getEncoding());
}
} catch(UnsupportedEncodingException exp) {
throw new ClientException("SDK.UnsupportedEncoding", "Can not parse response due to un supported encoding.");
}
return stringContent;
}
private KscError readError(HttpResponse httpResponse, FormatType format) throws ClientException {
KscError error = new KscError();
String responseEndpoint= "Error";
Reader reader = ReaderFactory.createInstance(format);
UnmarshallerContext context = new UnmarshallerContext();
String stringContent = getResponseContent(httpResponse);
context.setResponseMap(reader.read(stringContent,responseEndpoint));
return error.getInstance(context);
}
public boolean isAutoRetry() {
return autoRetry;
}
public void setAutoRetry(boolean autoRetry) {
this.autoRetry = autoRetry;
}
public int getMaxRetryNumber() {
return maxRetryNumber;
}
public void setMaxRetryNumber(int maxRetryNumber) {
this.maxRetryNumber = maxRetryNumber;
}
public void setUrlTestFlag(boolean flag) {
this.urlTestFlag = flag;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy