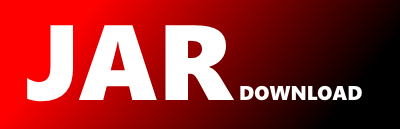
com.ksyun.api.sdk.kec.model.eip.DescribeAddressesRequest Maven / Gradle / Ivy
package com.ksyun.api.sdk.kec.model.eip;
import java.io.Serializable;
import com.ksc.KscWebServiceRequest;
import com.ksc.Request;
import com.ksc.model.DryRunSupportedRequest;
import com.ksc.model.Filter;
import com.ksc.util.json.Jackson;
import com.ksyun.api.sdk.kec.transform.eip.DescribeAddressesRequestMarshaller;
/**
*
* Contains the parameters for DescribeAddresses.
*
*/
public class DescribeAddressesRequest extends KscWebServiceRequest implements
Serializable, Cloneable,
DryRunSupportedRequest {
public DescribeAddressesRequest() {
initParam("com.ksyun.api.sdk.kec.KSC%sClient", "describeAddresses", "eip", "2016-03-04",this.getClass());
}
/**
*
*/
private static final long serialVersionUID = -3126063320884833699L;
/**
*
* One or more filters. Filter names and values are case-sensitive.
*
*
* -
*
* association-id
- The association ID for the address.
*
*
* -
*
* instance-type
- instance-type, if any.
*
*
* -
*
* network-interface-id
- The ID of the network interface that
* the address is associated with, if any.
*
*
* -
*
* internet-gateway-id
- internet-gateway-id.
*
*
*
*/
private com.ksc.internal.SdkInternalList filters;
/**
*
* One or more allocation IDs.
*
*
* Default: Describes all your Elastic IP addresses.
*
*/
private com.ksc.internal.SdkInternalList allocationIds;
private Integer maxResults;
private String nextToken;
public java.util.List getFilters() {
if (filters == null) {
filters = new com.ksc.internal.SdkInternalList();
}
return filters;
}
public void setFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
return;
}
this.filters = new com.ksc.internal.SdkInternalList(filters);
}
/**
*
* One or more filters. Filter names and values are case-sensitive.
*
*
* -
*
* association-id
- The association ID for the address.
*
*
* -
*
* instance-type
- instance-type, if any.
*
*
* -
*
* network-interface-id
- The ID of the network interface that
* the address is associated with, if any.
*
*
* -
*
* internet-gateway-id
- internet-gateway-id.
*
*
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setFilters(java.util.Collection)} or
* {@link #withFilters(java.util.Collection)} if you want to override the
* existing values.
*
*
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public DescribeAddressesRequest withFilters(Filter... filters) {
if (this.filters == null) {
setFilters(new com.ksc.internal.SdkInternalList(
filters.length));
}
for (Filter ele : filters) {
this.filters.add(ele);
}
return this;
}
/**
*
* One or more filters. Filter names and values are case-sensitive.
*
*
* -
*
* association-id
- The association ID for the address.
*
*
* -
*
* instance-type
- instance-type, if any.
*
*
* -
*
* network-interface-id
- The ID of the network interface that
* the address is associated with, if any.
*
*
* -
*
* internet-gateway-id
- internet-gateway-id.
*
*
*
*/
public DescribeAddressesRequest withFilters(
java.util.Collection filters) {
setFilters(filters);
return this;
}
/**
*
* One or more allocation IDs.
*
*
* Default: Describes all your Elastic IP addresses.
*
*
* @return One or more allocation IDs.
*
* Default: Describes all your Elastic IP addresses.
*/
public java.util.List getAllocationIds() {
if (allocationIds == null) {
allocationIds = new com.ksc.internal.SdkInternalList();
}
return allocationIds;
}
/**
*
* One or more allocation IDs.
*
*
* Default: Describes all your Elastic IP addresses.
*
*
* @param allocationIds
* One or more allocation IDs.
*
* Default: Describes all your Elastic IP addresses.
*/
public void setAllocationIds(java.util.Collection allocationIds) {
if (allocationIds == null) {
this.allocationIds = null;
return;
}
this.allocationIds = new com.ksc.internal.SdkInternalList(
allocationIds);
}
/**
*
* One or more allocation IDs.
*
*
* Default: Describes all your Elastic IP addresses.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setAllocationIds(java.util.Collection)} or
* {@link #withAllocationIds(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param allocationIds
* One or more allocation IDs.
*
* Default: Describes all your Elastic IP addresses.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public DescribeAddressesRequest withAllocationIds(String... allocationIds) {
if (this.allocationIds == null) {
setAllocationIds(new com.ksc.internal.SdkInternalList(
allocationIds.length));
}
for (String ele : allocationIds) {
this.allocationIds.add(ele);
}
return this;
}
/**
*
* One or more allocation IDs.
*
*
* Default: Describes all your Elastic IP addresses.
*
*
* @param allocationIds
* One or more allocation IDs.
*
* Default: Describes all your Elastic IP addresses.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public DescribeAddressesRequest withAllocationIds(
java.util.Collection allocationIds) {
setAllocationIds(allocationIds);
return this;
}
public Integer getMaxResults() {
return maxResults;
}
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
public String getNextToken() {
return nextToken;
}
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
* This method is intended for internal use only. Returns the marshaled
* request configured with additional parameters to enable operation
* dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new DescribeAddressesRequestMarshaller()
.marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
return Jackson.toJsonString(this);
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DescribeAddressesRequest == false)
return false;
DescribeAddressesRequest other = (DescribeAddressesRequest) obj;
if (other.getFilters() == null ^ this.getFilters() == null)
return false;
if (other.getFilters() != null
&& other.getFilters().equals(this.getFilters()) == false)
return false;
if (other.getAllocationIds() == null ^ this.getAllocationIds() == null)
return false;
if (other.getAllocationIds() != null
&& other.getAllocationIds().equals(this.getAllocationIds()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getFilters() == null) ? 0 : getFilters().hashCode());
hashCode = prime
* hashCode
+ ((getAllocationIds() == null) ? 0 : getAllocationIds()
.hashCode());
return hashCode;
}
@Override
public DescribeAddressesRequest clone() {
return (DescribeAddressesRequest) super.clone();
}
}