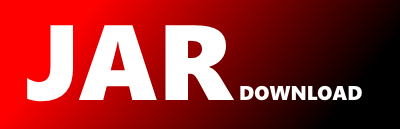
com.ksyun.api.sdk.request.KscRequest Maven / Gradle / Ivy
package com.ksyun.api.sdk.request;
import java.io.UnsupportedEncodingException;
import java.security.InvalidKeyException;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.Map.Entry;
import com.ksyun.api.sdk.auth.Credential;
import com.ksyun.api.sdk.auth.ISignatureComposer;
import com.ksyun.api.sdk.auth.ISigner;
import com.ksyun.api.sdk.auth.KscURLEncoder;
import com.ksyun.api.sdk.auth.RpcSignatureComposer;
import com.ksyun.api.sdk.http.FormatType;
import com.ksyun.api.sdk.http.MethodType;
import com.ksyun.api.sdk.http.ProtocolType;
import com.ksyun.api.sdk.regions.ProductDomain;
import com.ksyun.api.sdk.reponse.KscResponse;
public abstract class KscRequest extends HttpRequest {
public KscRequest(String product) {
super(product);
initialize();
}
public KscRequest(String product, String version) {
super(product);
this.setVersion(version);
initialize();
}
public KscRequest(String product, String version, String action) {
super(product);
this.setVersion(version);
this.setActionName(action);
initialize();
}
public KscRequest(String product, String version, String action, String locationProduct) {
super(product);
this.setVersion(version);
this.setActionName(action);
this.setLocationProduct(locationProduct);
initialize();
}
public KscRequest(String product, String version, String action, String locationProduct, String endpointType) {
super(product);
this.setVersion(version);
this.setActionName(action);
this.setLocationProduct(locationProduct);
this.setEndpointType(endpointType);
initialize();
}
private void initialize() {
this.setMethod(MethodType.GET);
this.setAcceptFormat(FormatType.XML);
this.composer = RpcSignatureComposer.getComposer();
}
public void setActionName(String actionName) {
this.actionName = actionName;
this.putQueryParameter("Action", actionName);
}
public void setVersion(String version) {
this.version = version;
this.putQueryParameter("Version", version);
}
public void setSecurityToken(String securityToken) {
this.securityToken = securityToken;
this.putQueryParameter("SecurityToken", securityToken);
}
public void setAcceptFormat(FormatType acceptFormat) {
this.acceptFormat = acceptFormat;
this.putQueryParameter("Format", acceptFormat.toString());
}
public String composeUrl(String endpoint, Map queries) throws UnsupportedEncodingException {
Map mapQueries = (queries == null) ? this.getQueryParameters() : queries;
StringBuilder urlBuilder = new StringBuilder("");
urlBuilder.append(this.getProtocol().toString());
urlBuilder.append("://").append(endpoint);
if (-1 == urlBuilder.indexOf("?")) {
urlBuilder.append("/?");
}
String query = concatQueryString(mapQueries);
return urlBuilder.append(query).toString();
}
public HttpRequest signRequest(ISigner signer, Credential credential, FormatType format, ProductDomain domain)
throws InvalidKeyException, IllegalStateException, UnsupportedEncodingException {
Map imutableMap = new HashMap(this.getQueryParameters());
if (null != signer && null != credential) {
String accessKeyId = credential.getAccessKeyId();
String accessSecret = credential.getAccessSecret();
imutableMap = this.composer.refreshSignParameters(this.getQueryParameters(), signer, accessKeyId, format);
imutableMap.put("RegionId", getRegionId());
String strToSign = this.composer.composeStringToSign(this.getMethod(), null, signer, imutableMap, null,
null);
String signature = signer.signString(strToSign, accessSecret + "&");
imutableMap.put("Signature", signature);
}
setUrl(this.composeUrl(domain.getDomianName(), imutableMap));
return this;
}
private String version = null;
private String product = null;
private String actionName = null;
private String regionId = null;
private String securityToken = null;
private FormatType acceptFormat = null;
protected ISignatureComposer composer = null;
private ProtocolType protocol = ProtocolType.HTTP;
private final Map queryParameters = new HashMap();
private final Map domainParameters = new HashMap();
private String locationProduct;
private String endpointType;
public String getLocationProduct() {
return locationProduct;
}
public void setLocationProduct(String locationProduct) {
this.locationProduct = locationProduct;
putQueryParameter("ServiceCode", locationProduct);
}
public String getEndpointType() {
return endpointType;
}
public void setEndpointType(String endpointType) {
this.endpointType = endpointType;
putQueryParameter("Type", endpointType);
}
public String getActionName() {
return actionName;
}
public String getProduct() {
return product;
}
public ProtocolType getProtocol() {
return protocol;
}
public void setProtocol(ProtocolType protocol) {
this.protocol = protocol;
}
public Map getQueryParameters() {
return Collections.unmodifiableMap(queryParameters);
}
public void putQueryParameter(String name, K value) {
setParameter(this.queryParameters, name, value);
}
protected void putQueryParameter(String name, String value) {
setParameter(this.queryParameters, name, value);
}
public Map getDomainParameters() {
return Collections.unmodifiableMap(domainParameters);
}
protected void putDomainParameter(String name, Object value) {
setParameter(this.domainParameters, name, value);
}
protected void putDomainParameter(String name, String value) {
setParameter(this.domainParameters, name, value);
}
protected void setParameter(Map map, String name, K value) {
if (null == map || null == name || null == value){
return;
}
map.put(name,String.valueOf(value));
}
public String getVersion() {
return version;
}
public FormatType getAcceptFormat() {
return acceptFormat;
}
public String getRegionId() {
return regionId;
}
public void setRegionId(String regionId) {
this.regionId = regionId;
}
public String getSecurityToken() {
return securityToken;
}
public static String concatQueryString(Map parameters)
throws UnsupportedEncodingException {
if (null == parameters)
return null;
StringBuilder urlBuilder = new StringBuilder("");
for(Entry entry : parameters.entrySet()){
String key = entry.getKey();
String val = entry.getValue();
urlBuilder.append(KscURLEncoder.encode(key));
if (val != null){
urlBuilder.append("=").append(KscURLEncoder.encode(val));
}
urlBuilder.append("&");
}
int strIndex = urlBuilder.length();
if (parameters.size() > 0)
urlBuilder.deleteCharAt(strIndex - 1);
return urlBuilder.toString();
}
public abstract Class getResponseClass();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy