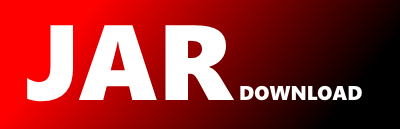
com.ksyun.api.sdk.http.ObjectPlaceholderResolver Maven / Gradle / Ivy
package com.ksyun.api.sdk.http;
import java.lang.reflect.Method;
import java.util.HashMap;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.util.PropertyPlaceholderHelper.PlaceholderResolver;
import org.springframework.util.ReflectionUtils;
import org.springframework.util.StringUtils;
public class ObjectPlaceholderResolver implements PlaceholderResolver {
private static final Logger logger = LoggerFactory.getLogger(ObjectPlaceholderResolver.class);
private Map placeholders;
public ObjectPlaceholderResolver(Object... objs) {
this.placeholders = new HashMap();
this.findGetMethods(objs);
}
@Override
public String resolvePlaceholder(String placeholder_name) {
Object obj = this.placeholders.get(placeholder_name);
if (obj == null) {
throw new IllegalStateException(String.format("can't resolve placeholder %s from %s property",
placeholder_name, obj));
}
String get_method_name = String.format("get%s", StringUtils.capitalize(placeholder_name));
Method m = ReflectionUtils.findMethod(obj.getClass(), get_method_name);
Object v = ReflectionUtils.invokeMethod(m, obj);
if (v == null || StringUtils.isEmpty(v.toString())) {
throw new IllegalStateException(String.format("can't resolve placeholder %s from %s property.",
placeholder_name, obj));
}
String value = v.toString();
logger.debug("resolve placeholder {}={} from {} property", placeholder_name, value, obj);
return value;
}
private void findGetMethods(Object... objs) {
for (Object obj : objs) {
Method[] methods = obj.getClass().getMethods();
for (Method m : methods) {
String method_name = m.getName();
if (method_name.startsWith("get")) {
String t = method_name.substring(3);
String placeholder = StringUtils.uncapitalize(t);
this.placeholders.put(placeholder, obj);
}
}
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy