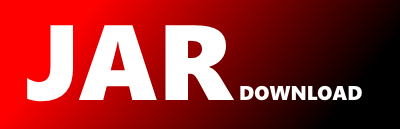
com.ksyun.api.sdk.kec.KSCEIPClient Maven / Gradle / Ivy
package com.ksyun.api.sdk.kec;
import java.util.ArrayList;
import java.util.List;
import org.w3c.dom.Node;
import com.ksc.ClientConfiguration;
import com.ksc.ClientConfigurationFactory;
import com.ksc.KscServiceException;
import com.ksc.KscWebServiceClient;
import com.ksc.KscWebServiceRequest;
import com.ksc.KscWebServiceResponse;
import com.ksc.Request;
import com.ksc.Response;
import com.ksc.auth.AWSCredentialsProvider;
import com.ksc.auth.DefaultAWSCredentialsProviderChain;
import com.ksc.auth.credentials.AWSCredentials;
import com.ksc.http.DefaultErrorResponseHandler;
import com.ksc.http.ExecutionContext;
import com.ksc.http.HttpResponseHandler;
import com.ksc.http.StaxResponseHandler;
import com.ksc.internal.StaticCredentialsProvider;
import com.ksc.metrics.RequestMetricCollector;
import com.ksc.transform.LegacyErrorUnmarshaller;
import com.ksc.transform.StandardErrorUnmarshaller;
import com.ksc.transform.Unmarshaller;
import com.ksc.util.CredentialUtils;
import com.ksc.util.KscRequestMetrics;
import com.ksc.util.KscRequestMetrics.Field;
import com.ksyun.api.sdk.kec.model.eip.DescribeAddressesRequest;
import com.ksyun.api.sdk.kec.model.eip.DescribeAddressesResult;
import com.ksyun.api.sdk.kec.transform.eip.DescribeAddressesRequestMarshaller;
import com.ksyun.api.sdk.kec.transform.eip.DescribeAddressesResultStaxUnmarshaller;
public class KSCEIPClient extends KscWebServiceClient implements KSCEIP{
/** Provider for AWS credentials. */
private AWSCredentialsProvider kscCredentialsProvider;
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "eip";
/** The region metadata service name for computing region endpoints. */
private static final String DEFAULT_ENDPOINT_PREFIX = "eip";
/**
* Client configuration factory providing ClientConfigurations tailored to
* this client
*/
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
/**
* List of exception unmarshallers for all modeled exceptions
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
/**
* Constructs a new client to invoke service methods on EIP. A credentials
* provider chain will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the EIP metadata
* service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public KSCEIPClient() {
this(new DefaultAWSCredentialsProviderChain(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on EIP. A credentials
* provider chain will be used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the EIP metadata
* service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to KSC (ex: proxy settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public KSCEIPClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on EIP using the
* specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
*/
public KSCEIPClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on EIP using the
* specified AWS account credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to EIP (ex: proxy settings, retry counts, etc.).
*/
public KSCEIPClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.kscCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on EIP using the
* specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with KSC services.
*/
public KSCEIPClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on EIP using the
* specified AWS account credentials provider and client configuration
* options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with KSC services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to EIP (ex: proxy settings, retry counts, etc.).
*/
public KSCEIPClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on EIP using the
* specified KSC account credentials provider, client configuration options,
* and request metric collector.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with KSC services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to EIP (ex: proxy settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
*/
public KSCEIPClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.kscCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
exceptionUnmarshallers.add(new StandardErrorUnmarshaller());
exceptionUnmarshallers.add(new LegacyErrorUnmarshaller());
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(DEFAULT_ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("http://eip.cn-beijing-6.api.ksyun.com");
}
@Override
public DescribeAddressesResult describeAddresses() {
return this.describeAddresses(new DescribeAddressesRequest());
}
@Override
public DescribeAddressesResult describeAddresses(DescribeAddressesRequest describeAddressesRequest) {
ExecutionContext executionContext = createExecutionContext(describeAddressesRequest);
KscRequestMetrics kscRequestMetrics = executionContext.getKscRequestMetrics();
kscRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
kscRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAddressesRequestMarshaller()
.marshall(super.beforeMarshalling(describeAddressesRequest));
// Binds the request metrics to the current request.
request.setKscRequestMetrics(kscRequestMetrics);
} finally {
kscRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeAddressesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getKscResponse();
} finally {
endClientExecution(kscRequestMetrics, request, response);
}
}
/**
* Normal invoke with authentication. Credentials are required and may be
* overriden at the request level.
**/
private Response invoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
executionContext.setCredentialsProvider(
CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), kscCredentialsProvider));
return doInvoke(request, responseHandler, executionContext);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack
* thereof) have been configured in the ExecutionContext beforehand.
**/
private Response doInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
}