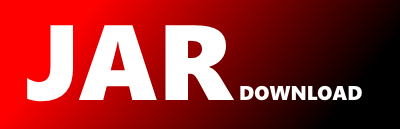
com.ksyun.api.sdk.kec.model.DescribeInstancesRequest Maven / Gradle / Ivy
package com.ksyun.api.sdk.kec.model;
import java.io.Serializable;
import com.ksc.KscWebServiceRequest;
import com.ksc.Request;
import com.ksc.model.DryRunSupportedRequest;
import com.ksc.model.Filter;
import com.ksyun.api.sdk.kec.transform.DescribeInstancesRequestMarshaller;
import lombok.EqualsAndHashCode;
import lombok.ToString;
/**
*
* Contains the parameters for DescribeInstances.
*
*/
@ToString
@EqualsAndHashCode(callSuper = false)
@SuppressWarnings("serial")
public class DescribeInstancesRequest extends KscWebServiceRequest implements
Serializable, Cloneable,
DryRunSupportedRequest {
public DescribeInstancesRequest(){
initParam("com.ksyun.api.sdk.kec.KSC%sClient", "describeInstances", "kec", "2016-03-04",this.getClass());
}
/**
*
* One or more instance IDs.
*
*
* Default: Describes all your instances.
*
*/
private com.ksc.internal.SdkInternalList instanceIds;
/**
*
* One or more filters.
*
*
* -
*
* instance-id
- instance-id
*
*
* -
*
* subnet-id
- subnet-id
*
*
* -
*
* vpc-id
- vpc-id
*
*
*/
private com.ksc.internal.SdkInternalList filters;
/**
*
* The token to request the next page of results.
*
*/
private String nextToken;
/**
*
* The maximum number of results to return in a single call. To retrieve the
* remaining results, make another call with the returned
* NextToken
value. This value can be between 5 and 1000. You
* cannot specify this parameter and the instance IDs parameter or tag
* filters in the same call.
*
*/
private Integer maxResults;
private Integer marker;
private String Search;
/**
*
* One or more instance IDs.
*
*
* Default: Describes all your instances.
*
*
* @return One or more instance IDs.
*
* Default: Describes all your instances.
*/
public java.util.List getInstanceIds() {
if (instanceIds == null) {
instanceIds = new com.ksc.internal.SdkInternalList();
}
return instanceIds;
}
/**
*
* One or more instance IDs.
*
*
* Default: Describes all your instances.
*
*
* @param instanceIds
* One or more instance IDs.
*
* Default: Describes all your instances.
*/
public void setInstanceIds(java.util.Collection instanceIds) {
if (instanceIds == null) {
this.instanceIds = null;
return;
}
this.instanceIds = new com.ksc.internal.SdkInternalList(
instanceIds);
}
/**
*
* One or more instance IDs.
*
*
* Default: Describes all your instances.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setInstanceIds(java.util.Collection)} or
* {@link #withInstanceIds(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param instanceIds
* One or more instance IDs.
*
* Default: Describes all your instances.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public DescribeInstancesRequest withInstanceIds(String... instanceIds) {
if (this.instanceIds == null) {
setInstanceIds(new com.ksc.internal.SdkInternalList(
instanceIds.length));
}
for (String ele : instanceIds) {
this.instanceIds.add(ele);
}
return this;
}
/**
*
* One or more instance IDs.
*
*
* Default: Describes all your instances.
*
*
* @param instanceIds
* One or more instance IDs.
*
* Default: Describes all your instances.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public DescribeInstancesRequest withInstanceIds(
java.util.Collection instanceIds) {
setInstanceIds(instanceIds);
return this;
}
/**
*
* One or more filters.
*
*
* -
*
* instance-id
- instance-id
*
*
* -
*
* subnet-id
- subnet-id
*
*
* -
*
* vpc-id
- vpc-id
*
*
*/
public java.util.List getFilters() {
if (filters == null) {
filters = new com.ksc.internal.SdkInternalList();
}
return filters;
}
public void setFilters(java.util.Collection filters) {
if (filters == null) {
this.filters = null;
return;
}
this.filters = new com.ksc.internal.SdkInternalList(
filters);
}
public DescribeInstancesRequest withFilters(Filter... filters) {
if (this.filters == null) {
setFilters(new com.ksc.internal.SdkInternalList(
filters.length));
}
for (Filter ele : filters) {
this.filters.add(ele);
}
return this;
}
public DescribeInstancesRequest withFilters(
java.util.Collection filters) {
setFilters(filters);
return this;
}
/**
*
* The token to request the next page of results.
*
*
* @param nextToken
* The token to request the next page of results.
*/
public void setNextToken(String nextToken) {
this.nextToken = nextToken;
}
/**
*
* The token to request the next page of results.
*
*
* @return The token to request the next page of results.
*/
public String getNextToken() {
return this.nextToken;
}
/**
*
* The token to request the next page of results.
*
*
* @param nextToken
* The token to request the next page of results.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public DescribeInstancesRequest withNextToken(String nextToken) {
setNextToken(nextToken);
return this;
}
/**
*
* The maximum number of results to return in a single call. To retrieve the
* remaining results, make another call with the returned
* NextToken
value. This value can be between 5 and 1000. You
* cannot specify this parameter and the instance IDs parameter or tag
* filters in the same call.
*
*
* @param maxResults
* The maximum number of results to return in a single call. To
* retrieve the remaining results, make another call with the
* returned NextToken
value. This value can be
* between 5 and 1000. You cannot specify this parameter and the
* instance IDs parameter or tag filters in the same call.
*/
public void setMaxResults(Integer maxResults) {
this.maxResults = maxResults;
}
/**
*
* The maximum number of results to return in a single call. To retrieve the
* remaining results, make another call with the returned
* NextToken
value. This value can be between 5 and 1000. You
* cannot specify this parameter and the instance IDs parameter or tag
* filters in the same call.
*
*
* @return The maximum number of results to return in a single call. To
* retrieve the remaining results, make another call with the
* returned NextToken
value. This value can be between
* 5 and 1000. You cannot specify this parameter and the instance
* IDs parameter or tag filters in the same call.
*/
public Integer getMaxResults() {
return this.maxResults;
}
/**
*
* The maximum number of results to return in a single call. To retrieve the
* remaining results, make another call with the returned
* NextToken
value. This value can be between 5 and 1000. You
* cannot specify this parameter and the instance IDs parameter or tag
* filters in the same call.
*
*
* @param maxResults
* The maximum number of results to return in a single call. To
* retrieve the remaining results, make another call with the
* returned NextToken
value. This value can be
* between 5 and 1000. You cannot specify this parameter and the
* instance IDs parameter or tag filters in the same call.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public DescribeInstancesRequest withMaxResults(Integer maxResults) {
setMaxResults(maxResults);
return this;
}
/**
* This method is intended for internal use only. Returns the marshaled
* request configured with additional parameters to enable operation
* dry-run.
*/
@Override
public Request getDryRunRequest() {
Request request = new DescribeInstancesRequestMarshaller()
.marshall(this);
request.addParameter("DryRun", Boolean.toString(true));
return request;
}
@Override
public DescribeInstancesRequest clone() {
return (DescribeInstancesRequest) super.clone();
}
public Integer getMarker() {
return marker;
}
public void setMarker(Integer marker) {
this.marker = marker;
}
public String getSearch() {
return Search;
}
public void setSearch(String search) {
Search = search;
}
}