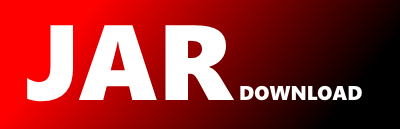
com.github.kubatatami.judonetworking.Request Maven / Gradle / Ivy
package com.github.kubatatami.judonetworking;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
class Request implements Runnable, Comparable, ProgressObserver, RequestInterface {
private Integer id;
private final EndpointImplementation rpc;
private CallbackInterface
© 2015 - 2025 Weber Informatics LLC | Privacy Policy