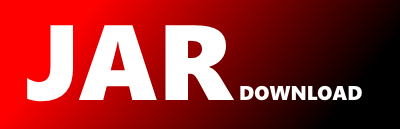
com.github.kunalk16.excel.objectmapper.extractor.FieldsFromClassExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lightExcelReader Show documentation
Show all versions of lightExcelReader Show documentation
A lightweight Java framework to read .xlsx excel files.
package com.github.kunalk16.excel.objectmapper.extractor;
import com.github.kunalk16.excel.utils.logger.ExcelReaderLogger;
import java.lang.annotation.Annotation;
import java.lang.reflect.Field;
import java.util.*;
import java.util.function.Function;
public class FieldsFromClassExtractor implements Function, Map> {
private final Class annotationClass;
public FieldsFromClassExtractor(Class annotationClass) {
this.annotationClass = annotationClass;
}
@Override
public Map apply(Class> modelClass) {
List> allClasses = getAllClasses(modelClass);
Map fieldsByName = new HashMap<>();
allClasses.stream()
.map(Class::getDeclaredFields)
.map(Arrays::asList)
.flatMap(Collection::stream)
.filter(this::isFieldAnnotated)
.forEach(field -> storeFieldByName(field, fieldsByName));
return fieldsByName;
}
private List> getAllClasses(Class> clazz) {
List> allClasses = new LinkedList<>();
Class> currentClass = clazz;
while (currentClass != Object.class) {
allClasses.add(currentClass);
currentClass = currentClass.getSuperclass();
}
return allClasses;
}
private boolean isFieldAnnotated(Field field) {
return Objects.nonNull(field.getAnnotation(annotationClass));
}
private void storeFieldByName(Field field, Map fieldsByName) {
T annotation = field.getAnnotation(annotationClass);
try {
fieldsByName.put(annotation.getClass().getMethod("name").invoke(annotation).toString(), field);
} catch (Exception e) {
ExcelReaderLogger.getInstance().severe("Could not generate fields by name " + e.getLocalizedMessage());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy