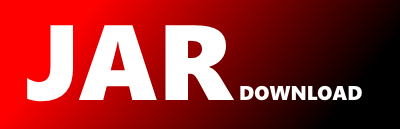
com.github.kunalk16.excel.objectmapper.extractor.ObjectFromRowExtractor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of lightExcelReader Show documentation
Show all versions of lightExcelReader Show documentation
A lightweight Java framework to read .xlsx excel files.
The newest version!
package com.github.kunalk16.excel.objectmapper.extractor;
import com.github.kunalk16.excel.model.user.Row;
import com.github.kunalk16.excel.utils.logger.ExcelReaderLogger;
import java.lang.reflect.Field;
import java.util.Map;
import java.util.Objects;
import java.util.function.Function;
public class ObjectFromRowExtractor implements Function {
private final Class modelClass;
private final Map fieldByName;
private final Map columnByHeaderName;
public ObjectFromRowExtractor(Class modelClass, Map fieldByName, Map columnByHeaderName) {
this.modelClass = modelClass;
this.fieldByName = fieldByName;
this.columnByHeaderName = columnByHeaderName;
}
@Override
public T apply(Row row) {
try {
T instance = modelClass.newInstance();
for (String fieldName : fieldByName.keySet()) {
if (fieldByName.containsKey(fieldName) && columnByHeaderName.containsKey(fieldName) &&
Objects.nonNull(row.getCellByColumn(columnByHeaderName.get(fieldName)))) {
Field field = getField(modelClass, fieldByName.get(fieldName).getName());
field.setAccessible(true);
field.set(instance, row.getCellByColumn(columnByHeaderName.get(fieldName)).getValue());
}
}
return instance;
} catch (Exception e) {
ExcelReaderLogger.getInstance().severe("Could not create instance of row object " + e.getLocalizedMessage());
return null;
}
}
private Field getField(Class> modelClass, String fieldName) throws Exception {
try {
return modelClass.getDeclaredField(fieldName);
} catch (Exception e) {
if (modelClass.getSuperclass() != Object.class) {
return getField(modelClass.getSuperclass(), fieldName);
}
throw e;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy