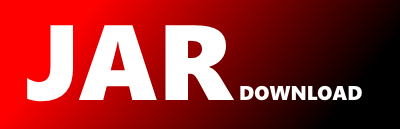
com.github.kzwang.osem.annotations.Indexable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of elasticsearch-osem Show documentation
Show all versions of elasticsearch-osem Show documentation
Object/Search Engine Mapping for ElasticSearch
The newest version!
package com.github.kzwang.osem.annotations;
import com.fasterxml.jackson.databind.JsonDeserializer;
import com.fasterxml.jackson.databind.JsonSerializer;
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
/**
* Root object type
*
* @see Root Object Type
*/
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.TYPE)
public @interface Indexable {
/**
* Index type name, default to class name in lower_underscore format
*/
String name() default "";
/**
* "index_analyzer" field in mapping
*/
String indexAnalyzer() default "";
/**
* "search_analyzer" field in mapping
*/
String searchAnalyzer() default "";
/**
* "dynamic_date_formats" field in mapping
*/
String[] dynamicDateFormats() default {};
/**
* "date_detection" field in mapping
*/
DateDetectionEnum dateDetection() default DateDetectionEnum.NA;
/**
* "numeric_detection" field in mapping
*/
NumericDetectionEnum numericDetection() default NumericDetectionEnum.NA;
/**
* path of parent id field
*/
String parentPath() default "";
/**
* Class of the parent type
*/
Class parentClass() default void.class;
/**
* "store" in "_type"
*
* @see Mapping type field
*/
boolean typeFieldStore() default false;
/**
* "index" in "_type"
*
* @see Mapping type field
*/
IndexEnum typeFieldIndex() default IndexEnum.NA;
/**
* "enabled" in "_source"
*
* @see Mapping source field
*/
boolean sourceFieldEnabled() default true;
/**
* "compress" in "_source"
*
* @see Mapping source field compress
*/
boolean sourceFieldCompress() default false;
/**
* "compress_threshold" in "_source"
*
* @see Mapping source field compress
*/
String sourceFieldCompressThreshold() default "";
/**
* "includes" in "_source"
*
* @see Mapping source field include
*/
String[] sourceFieldIncludes() default {};
/**
* "excludes" in "_source"
*
* @see Mapping source field exclude
*/
String[] sourceFieldExcludes() default {};
/**
* "enabled" in "_all"
*
* @see Mapping all field
*/
boolean allFieldEnabled() default true;
/**
* "store" in "_all"
*
* @see Mapping all field
*/
boolean allFieldStore() default false;
/**
* "term_vector" in "_all"
*
* @see Mapping all field
*/
TermVectorEnum allFieldTermVector() default TermVectorEnum.NA;
/**
* "analyzer" in "_all"
*
* @see Mapping all field
*/
String allFieldAnalyzer() default "";
/**
* "index_analyzer" in "_all"
*
* @see Mapping all field
*/
String allFieldIndexAnalyzer() default "";
/**
* "search_analyzer" in "_all"
*
* @see Mapping all field
*/
String allFieldSearchAnalyzer() default "";
/**
* "path" in "_analyzer"
*
* @see Mapping analyzer field
*/
String analyzerFieldPath() default "";
/**
* "name" in "_boost"
*
* @see Mapping boost field
* @deprecated deprecated in ElasticSearch 1.0.0.rc1
*/
@Deprecated
String boostFieldName() default "";
/**
* "name" in "_boost"
*
* @see Mapping boost field
* @deprecated deprecated in ElasticSearch 1.0.0.rc1
*/
@Deprecated
double boostFieldNullValue() default Double.MIN_VALUE;
/**
* "store" in "_routing"
*
* @see Mapping routing field
*/
boolean routingFieldStore() default true;
/**
* "index" in "_routing"
*
* @see Mapping routing field
*/
IndexEnum routingFieldIndex() default IndexEnum.NA;
/**
* "required" in "_routing"
*
* @see Mapping routing field
*/
boolean routingFieldRequired() default false;
/**
* "path" in "_routing"
*
* @see Mapping routing field
*/
String routingFieldPath() default "";
/**
* "enabled" in "_index"
*
* @see Mapping index field
*/
boolean indexFieldEnabled() default false;
/**
* "enabled" in "_size"
*
* @see Mapping size field
*/
boolean sizeFieldEnabled() default false;
/**
* "store" in "_size"
*
* @see Mapping size field
*/
boolean sizeFieldStore() default false;
/**
* "enabled" in "_timestamp"
*
* @see Mapping timestamp field
*/
boolean timestampFieldEnabled() default false;
/**
* "store" in "_timestamp"
*
* @see Mapping timestamp field
*/
boolean timestampFieldStore() default false;
/**
* "index" in "_timestamp"
*
* @see Mapping timestamp field
*/
IndexEnum timestampFieldIndex() default IndexEnum.NA;
/**
* "path" in "_timestamp"
*
* @see Mapping timestamp field
*/
String timestampFieldPath() default "";
/**
* "format" in "_timestamp"
*
* @see Mapping timestamp field
*/
String timestampFieldFormat() default "";
/**
* "enabled" in "_ttl"
*
* @see Mapping ttl field
*/
boolean ttlFieldEnabled() default false;
/**
* "store" in "_ttl"
*
* @see Mapping ttl field
*/
boolean ttlFieldStore() default true;
/**
* "index" in "_ttl"
*
* @see Mapping ttl field
*/
IndexEnum ttlFieldIndex() default IndexEnum.NA;
/**
* "default" in "_timestamp"
*
* @see Mapping ttl field
*/
String ttlFieldDefault() default "";
/**
* Json DeSerializer class, must extend {@link JsonDeserializer}
*/
Class extends JsonDeserializer> deserializer() default JsonDeserializer.class;
/**
* Json Serializer class, must extend {@link JsonSerializer}
*/
Class extends JsonSerializer> serializer() default JsonSerializer.class;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy