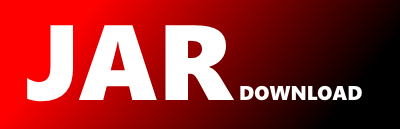
com.jladder.lang.LRUCache Maven / Gradle / Ivy
package com.jladder.lang;
import java.util.*;
public class LRUCache {
private static final float hashTableLoadFactor = 0.75f;
private LinkedHashMap map;
private int cacheSize;
/***
* 构造
* @param cacheSize 缓存大小
*/
public LRUCache(int cacheSize) {
this.cacheSize = cacheSize;
int hashTableCapacity = (int) Math.ceil(cacheSize / hashTableLoadFactor) + 1;
map = new LinkedHashMap(hashTableCapacity, hashTableLoadFactor,true) {
// (an anonymous inner class)
private static final long serialVersionUID = 1;
@Override
protected boolean removeEldestEntry(Map.Entry eldest) {
return size() > LRUCache.this.cacheSize;
}
};
}
public synchronized V get(K key) {
return map.get(key);
}
public synchronized void put(K key, V value) {
map.put(key, value);
}
public synchronized void clear() {
map.clear();
}
public synchronized void remove(String key){
map.remove(key);
}
public synchronized int usedEntries() {
return map.size();
}
public synchronized Collection> getAll() {
return new ArrayList>(map.entrySet());
}
public synchronized List getValues() {
return new ArrayList(map.values());
}
public void setCacheSize(int cacheSize) {
this.cacheSize = cacheSize;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy