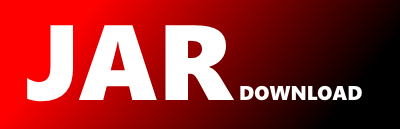
com.github.leanjaxrs.test.petstore_full.api.User Maven / Gradle / Ivy
package com.github.leanjaxrs.test.petstore_full.api;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnumValue;
import javax.xml.bind.annotation.XmlElement;
import java.util.Objects;
import java.util.Optional;
public class User {
@XmlElement(name="id")
private Long id;
@XmlElement(name="username")
private String username;
@XmlElement(name="firstName")
private String firstName;
@XmlElement(name="lastName")
private String lastName;
@XmlElement(name="email")
private String email;
@XmlElement(name="password")
private String password;
@XmlElement(name="phone")
private String phone;
@XmlElement(name="userStatus")
private Integer userStatus;
/**
* @return id
**/
public Long getId() {
return id;
}
/**
* @param id Set the id property.
*/
public void setId(Long id) {
this.id = id;
}
/**
* @return username
**/
public String getUsername() {
return username;
}
/**
* @param username Set the username property.
*/
public void setUsername(String username) {
this.username = username;
}
/**
* @return firstName
**/
public String getFirstName() {
return firstName;
}
/**
* @param firstName Set the firstName property.
*/
public void setFirstName(String firstName) {
this.firstName = firstName;
}
/**
* @return lastName
**/
public String getLastName() {
return lastName;
}
/**
* @param lastName Set the lastName property.
*/
public void setLastName(String lastName) {
this.lastName = lastName;
}
/**
* @return email
**/
public String getEmail() {
return email;
}
/**
* @param email Set the email property.
*/
public void setEmail(String email) {
this.email = email;
}
/**
* @return password
**/
public String getPassword() {
return password;
}
/**
* @param password Set the password property.
*/
public void setPassword(String password) {
this.password = password;
}
/**
* @return phone
**/
public String getPhone() {
return phone;
}
/**
* @param phone Set the phone property.
*/
public void setPhone(String phone) {
this.phone = phone;
}
/**
* User Status
* @return userStatus
**/
public Integer getUserStatus() {
return userStatus;
}
/**
* @param userStatus Set the userStatus property.
*/
public void setUserStatus(Integer userStatus) {
this.userStatus = userStatus;
}
/**
* Creates a builder for User instances
* @return the new User builder.
*/
public static Builder builder() {
return new Builder();
}
/**
* A builder class for building User objects with a fluent API. So to
* build an instance, use a pattern like this:
*
* User instance = User.builder()
* // set properties of the new instance...
* .id(idValue)
* .username(usernameValue)
* .firstName(firstNameValue)
* .lastName(lastNameValue)
* .email(emailValue)
* .password(passwordValue)
* .phone(phoneValue)
* .userStatus(userStatusValue)
* // ...and finally build the object!
* .build();
*
*/
public static class Builder {
private final User o = new User();
/**
* Finish building the instance and return it
* @return the newly built User instance
*/
public User build() {
return o;
}
/**
* Set the id property
* @param id value to set
* @return This builder
*/
public Builder id(Long id) {
o.setId(id);
return this;
}
/**
* Set the id property if the given optional is present.
* @param idOpt optional value to set, if present
* @return This builder
*/
public Builder id(Optional idOpt) {
idOpt.ifPresent(this::id);
return this;
}
/**
* Set the username property
* @param username value to set
* @return This builder
*/
public Builder username(String username) {
o.setUsername(username);
return this;
}
/**
* Set the username property if the given optional is present.
* @param usernameOpt optional value to set, if present
* @return This builder
*/
public Builder username(Optional usernameOpt) {
usernameOpt.ifPresent(this::username);
return this;
}
/**
* Set the firstName property
* @param firstName value to set
* @return This builder
*/
public Builder firstName(String firstName) {
o.setFirstName(firstName);
return this;
}
/**
* Set the firstName property if the given optional is present.
* @param firstNameOpt optional value to set, if present
* @return This builder
*/
public Builder firstName(Optional firstNameOpt) {
firstNameOpt.ifPresent(this::firstName);
return this;
}
/**
* Set the lastName property
* @param lastName value to set
* @return This builder
*/
public Builder lastName(String lastName) {
o.setLastName(lastName);
return this;
}
/**
* Set the lastName property if the given optional is present.
* @param lastNameOpt optional value to set, if present
* @return This builder
*/
public Builder lastName(Optional lastNameOpt) {
lastNameOpt.ifPresent(this::lastName);
return this;
}
/**
* Set the email property
* @param email value to set
* @return This builder
*/
public Builder email(String email) {
o.setEmail(email);
return this;
}
/**
* Set the email property if the given optional is present.
* @param emailOpt optional value to set, if present
* @return This builder
*/
public Builder email(Optional emailOpt) {
emailOpt.ifPresent(this::email);
return this;
}
/**
* Set the password property
* @param password value to set
* @return This builder
*/
public Builder password(String password) {
o.setPassword(password);
return this;
}
/**
* Set the password property if the given optional is present.
* @param passwordOpt optional value to set, if present
* @return This builder
*/
public Builder password(Optional passwordOpt) {
passwordOpt.ifPresent(this::password);
return this;
}
/**
* Set the phone property
* @param phone value to set
* @return This builder
*/
public Builder phone(String phone) {
o.setPhone(phone);
return this;
}
/**
* Set the phone property if the given optional is present.
* @param phoneOpt optional value to set, if present
* @return This builder
*/
public Builder phone(Optional phoneOpt) {
phoneOpt.ifPresent(this::phone);
return this;
}
/**
* Set the userStatus property
* @param userStatus value to set
* @return This builder
*/
public Builder userStatus(Integer userStatus) {
o.setUserStatus(userStatus);
return this;
}
/**
* Set the userStatus property if the given optional is present.
* @param userStatusOpt optional value to set, if present
* @return This builder
*/
public Builder userStatus(Optional userStatusOpt) {
userStatusOpt.ifPresent(this::userStatus);
return this;
}
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
User user = (User) o;
return Objects.equals(id, user.id) &&
Objects.equals(username, user.username) &&
Objects.equals(firstName, user.firstName) &&
Objects.equals(lastName, user.lastName) &&
Objects.equals(email, user.email) &&
Objects.equals(password, user.password) &&
Objects.equals(phone, user.phone) &&
Objects.equals(userStatus, user.userStatus);
}
@Override
public int hashCode() {
return Objects.hash(id, username, firstName, lastName, email, password, phone, userStatus);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class User {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" username: ").append(toIndentedString(username)).append("\n");
sb.append(" firstName: ").append(toIndentedString(firstName)).append("\n");
sb.append(" lastName: ").append(toIndentedString(lastName)).append("\n");
sb.append(" email: ").append(toIndentedString(email)).append("\n");
sb.append(" password: ").append(toIndentedString(password)).append("\n");
sb.append(" phone: ").append(toIndentedString(phone)).append("\n");
sb.append(" userStatus: ").append(toIndentedString(userStatus)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy