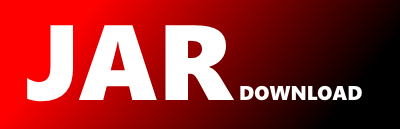
com.github.leeonky.dal.format.BaseFormatter Maven / Gradle / Ivy
package com.github.leeonky.dal.format;
import com.github.leeonky.dal.token.IllegalTypeException;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
public abstract class BaseFormatter implements Formatter {
private final Class inputType;
@SuppressWarnings("unchecked")
protected BaseFormatter() {
inputType = (Class) guessInputType(getClass().getGenericSuperclass());
}
public static Class> guessInputType(Type type) {
if (type instanceof ParameterizedType) {
ParameterizedType parameterizedType = (ParameterizedType) type;
if (parameterizedType.getRawType().equals(BaseFormatter.class))
return (Class>) parameterizedType.getActualTypeArguments()[0];
else
return guessInputType(parameterizedType.getRawType());
} else if (type instanceof Class)
return guessInputType(((Class) type).getGenericSuperclass());
throw new IllegalStateException("Cannot guess input type.");
}
public static R toValueOrThrowIllegalTypeException(T arg, ParseBlock parseBlock) {
try {
return parseBlock.run(arg);
} catch (Exception e) {
throw new IllegalTypeException();
}
}
@Override
public boolean isValidType(Object input) {
return inputType.isInstance(input);
}
@FunctionalInterface
public
interface ParseBlock {
R2 run(T2 t) throws Exception;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy