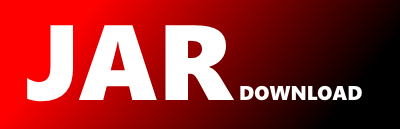
com.github.leeonky.dal.runtime.AutoMappingList Maven / Gradle / Ivy
package com.github.leeonky.dal.runtime;
import java.util.ArrayList;
import java.util.Collection;
import java.util.function.Function;
public class AutoMappingList extends ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy