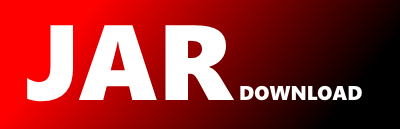
com.github.leeonky.dal.runtime.inspector.MapInspector Maven / Gradle / Ivy
package com.github.leeonky.dal.runtime.inspector;
import com.github.leeonky.dal.runtime.Data;
import com.github.leeonky.dal.util.TextUtil;
import com.github.leeonky.util.Classes;
import java.util.Map;
import java.util.Set;
import static java.util.stream.Collectors.joining;
public class MapInspector extends CacheableInspector {
public MapInspector(Data data) {
super(data);
}
@Override
public String cachedInspect(String path, InspectorCache cache) {
String type = type();
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy