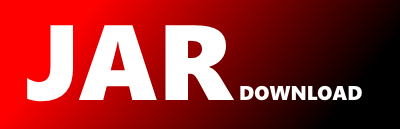
com.github.leeonky.dal.ast.node.SchemaComposeNode Maven / Gradle / Ivy
package com.github.leeonky.dal.ast.node;
import com.github.leeonky.dal.runtime.AssertionFailure;
import com.github.leeonky.dal.runtime.Data;
import com.github.leeonky.dal.runtime.IllegalTypeException;
import com.github.leeonky.dal.runtime.RuntimeContextBuilder.DALRuntimeContext;
import com.github.leeonky.dal.runtime.RuntimeException;
import java.util.List;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.stream.Collector;
import static java.lang.String.format;
import static java.util.stream.Collectors.joining;
import static java.util.stream.Collectors.toList;
public class SchemaComposeNode extends DALNode {
private final List schemas;
private final boolean isList;
public SchemaComposeNode(List schemas, boolean isList) {
this.schemas = schemas;
this.isList = isList;
}
@Override
public String inspect() {
Collector joining = isList ? joining(" / ", "[", "]") : joining(" / ");
return schemas.stream().map(SchemaNode::inspect).collect(joining);
}
public Data verify(DALNode input, DALRuntimeContext context) {
try {
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy