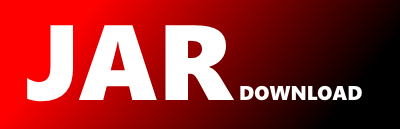
com.github.leeonky.dal.runtime.AssertionFailure Maven / Gradle / Ivy
package com.github.leeonky.dal.runtime;
import java.util.Set;
import java.util.regex.Pattern;
import static java.lang.String.format;
import static java.util.stream.Collectors.joining;
public class AssertionFailure extends DalException {
public AssertionFailure(String message, int position) {
super(message, position);
}
public static void assertUnexpectedFields(Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy