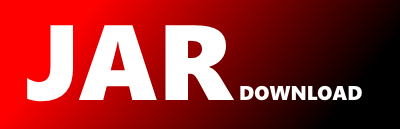
com.github.leeonky.dal.runtime.JavaClassPropertyAccessor Maven / Gradle / Ivy
package com.github.leeonky.dal.runtime;
import com.github.leeonky.util.BeanClass;
import com.github.leeonky.util.NoSuchAccessorException;
import java.util.LinkedHashSet;
import java.util.Objects;
import java.util.Set;
import static java.lang.String.format;
public class JavaClassPropertyAccessor implements PropertyAccessor {
private final BeanClass beanClass;
public JavaClassPropertyAccessor(BeanClass type) {
beanClass = type;
}
@Override
public Object getValue(T instance, Object property) {
try {
return beanClass.getPropertyValue(instance, (String) property);
} catch (NoSuchAccessorException ignore) {
throw new InvalidPropertyException(format("Method or property `%s` does not exist in `%s`", property, instance.getClass().getName()));
}
}
@Override
public Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy