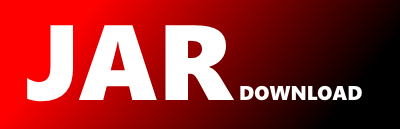
com.github.leeonky.dal.runtime.PartialProperties Maven / Gradle / Ivy
package com.github.leeonky.dal.runtime;
import java.util.LinkedHashSet;
import java.util.Optional;
import java.util.Set;
import java.util.stream.Collectors;
class PartialProperties {
final Object prefix;
final Data partialData;
final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy