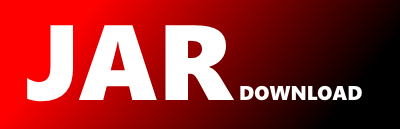
com.github.leeonky.dal.runtime.schema.Actual Maven / Gradle / Ivy
package com.github.leeonky.dal.runtime.schema;
import com.github.leeonky.dal.compiler.Compiler;
import com.github.leeonky.dal.format.Formatter;
import com.github.leeonky.dal.format.Type;
import com.github.leeonky.dal.format.Value;
import com.github.leeonky.dal.runtime.Data;
import com.github.leeonky.dal.runtime.SchemaAssertionFailure;
import com.github.leeonky.dal.type.Schema;
import com.github.leeonky.dal.type.SubType;
import com.github.leeonky.util.BeanClass;
import java.util.Objects;
import java.util.function.Function;
import java.util.stream.Stream;
import static com.github.leeonky.util.Classes.getClassName;
import static java.lang.String.format;
import static java.util.Optional.ofNullable;
import static java.util.stream.IntStream.range;
//TODO use ExpectActual build error message
public class Actual {
private final String property;
private final Data actual;
public Actual(String property, Data actual) {
this.property = property;
this.actual = actual;
}
public static Actual actual(Data data) {
return new Actual("", data);
}
public Actual sub(Object property) {
return new Actual(this.property + "." + property, actual.getValue(property));
}
public boolean isNull() {
return actual.isNull();
}
public Actual sub(Integer index) {
return new Actual(property + "[" + index + "]", actual.getValue(index));
}
private final static Compiler compiler = new Compiler();
@SuppressWarnings("unchecked")
public Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy