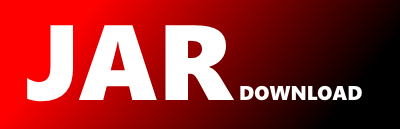
com.github.leeonky.dal.runtime.schema.Expect Maven / Gradle / Ivy
package com.github.leeonky.dal.runtime.schema;
import com.github.leeonky.dal.format.Formatter;
import com.github.leeonky.dal.format.Type;
import com.github.leeonky.dal.format.Value;
import com.github.leeonky.dal.runtime.RuntimeContextBuilder;
import com.github.leeonky.dal.type.AllowNull;
import com.github.leeonky.dal.type.Partial;
import com.github.leeonky.dal.type.Schema;
import com.github.leeonky.util.BeanClass;
import com.github.leeonky.util.Classes;
import com.github.leeonky.util.PropertyAccessor;
import com.github.leeonky.util.PropertyReader;
import java.util.LinkedHashSet;
import java.util.Map;
import java.util.Optional;
import java.util.Set;
import java.util.function.BiPredicate;
import java.util.stream.Stream;
import static com.github.leeonky.util.CollectionHelper.toStream;
import static java.lang.String.format;
import static java.lang.String.valueOf;
import static java.util.stream.Collectors.joining;
public class Expect {
protected final BeanClass
© 2015 - 2025 Weber Informatics LLC | Privacy Policy