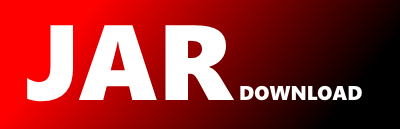
com.github.leeonky.util.BeanClass Maven / Gradle / Ivy
The newest version!
package com.github.leeonky.util;
import java.lang.annotation.Annotation;
import java.lang.reflect.Array;
import java.lang.reflect.Type;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
import static java.util.Arrays.asList;
import static java.util.Optional.ofNullable;
import static java.util.stream.Collectors.toList;
public class BeanClass {
private final static Map, BeanClass>> instanceCache = new ConcurrentHashMap<>();
private static Converter converter = Converter.getInstance();
private final TypeInfo typeInfo;
private final Class type;
protected BeanClass(Class type) {
this.type = Objects.requireNonNull(type);
typeInfo = TypeInfo.create(this);
}
@SuppressWarnings("unchecked")
public static BeanClass create(Class type) {
return (BeanClass) instanceCache.computeIfAbsent(type, BeanClass::new);
}
public static Optional cast(Object value, Class type) {
return ofNullable(value)
.filter(type::isInstance)
.map(type::cast);
}
public static BeanClass> create(GenericType type) {
if (!type.hasTypeArguments())
return create(type.getRawType());
return GenericBeanClass.create(type);
}
@SuppressWarnings("unchecked")
public static Class getClass(T instance) {
return (Class) Objects.requireNonNull(instance).getClass();
}
public static BeanClass createFrom(T instance) {
return create(getClass(instance));
}
public static Converter getConverter() {
return converter;
}
public static void setConverter(Converter converter) {
BeanClass.converter = converter;
}
public Class getType() {
return type;
}
public String getName() {
return type.getName();
}
public String getSimpleName() {
return type.getSimpleName();
}
public Map> getPropertyReaders() {
return typeInfo.getReaders();
}
public Map> getPropertyWriters() {
return typeInfo.getWriters();
}
public Object getPropertyValue(T bean, String property) {
return getPropertyReader(property).getValue(bean);
}
public PropertyReader getPropertyReader(String property) {
return typeInfo.getReader(property);
}
public BeanClass setPropertyValue(T bean, String property, Object value) {
getPropertyWriter(property).setValue(bean, value);
return this;
}
public PropertyWriter getPropertyWriter(String property) {
return typeInfo.getWriter(property);
}
public T newInstance(Object... args) {
return Classes.newInstance(type, args);
}
public Object createCollection(Collection> elements) {
return CollectionHelper.createCollection(elements, this);
}
public Object getPropertyChainValue(T object, String chain) {
return getPropertyChainValue(object, Property.toChainNodes(chain));
}
public Object getPropertyChainValue(T object, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy