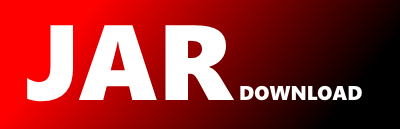
com.github.leeonky.util.CollectionDataPropertyWriter Maven / Gradle / Ivy
The newest version!
package com.github.leeonky.util;
import java.lang.reflect.Array;
import java.util.List;
import java.util.function.BiConsumer;
import static java.lang.Integer.parseInt;
class CollectionDataPropertyWriter extends DataPropertyAccessor implements PropertyWriter {
@SuppressWarnings("unchecked")
private final BiConsumer SETTER = (bean, value) -> {
Class type = getBeanType().getType();
int index = parseInt(getName());
if (type.isArray())
Array.set(bean, index, value);
else if (List.class.isAssignableFrom(type))
((List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy