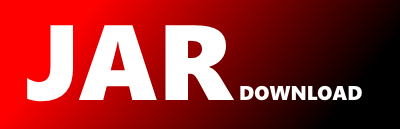
com.github.leeonky.util.CollectionHelper Maven / Gradle / Ivy
The newest version!
package com.github.leeonky.util;
import java.lang.reflect.Array;
import java.util.*;
import java.util.stream.Collectors;
import java.util.stream.IntStream;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import static com.github.leeonky.util.BeanClass.create;
public class CollectionHelper {
@SuppressWarnings("unchecked")
public static Stream toStream(Object collection) {
if (collection != null) {
Class> collectionType = collection.getClass();
if (collectionType.isArray())
return IntStream.range(0, Array.getLength(collection)).mapToObj(i -> (E) Array.get(collection, i));
else if (collection instanceof Iterable)
return StreamSupport.stream(((Iterable) collection).spliterator(), false);
else if (collection instanceof Stream)
return (Stream) collection;
}
throw new CannotToStreamException(collection);
}
public static T convert(Object collection, BeanClass toType) {
return convert(collection, toType, Converter.getInstance());
}
@SuppressWarnings("unchecked")
public static T convert(Object collection, BeanClass toType, Converter elementConverter) {
if (collection != null) {
Class> elementType = toType.getElementType().getType();
return (T) toType.createCollection((toStream(collection).map(o ->
elementConverter.convert(elementType, o))).collect(Collectors.toList()));
}
return null;
}
public static Object createCollection(Collection> elements, BeanClass> type) {
if (type.getType().isArray())
return createArray(elements, type);
if (type.getType().isInterface())
return createInterfaceCollection(elements, type);
if (Collection.class.isAssignableFrom(type.getType()))
return createClassCollection(elements, type);
throw new IllegalStateException(String.format("Cannot create instance of collection type %s", type.getName()));
}
@SuppressWarnings("unchecked")
private static Collection
© 2015 - 2024 Weber Informatics LLC | Privacy Policy