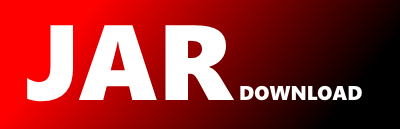
com.github.leeonky.util.Property Maven / Gradle / Ivy
The newest version!
package com.github.leeonky.util;
import java.util.Arrays;
import java.util.List;
import static java.util.stream.Collectors.toList;
public interface Property {
static List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy