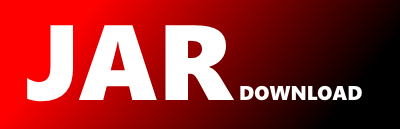
com.github.leeonky.util.Zipped Maven / Gradle / Ivy
The newest version!
package com.github.leeonky.util;
import java.util.Iterator;
import java.util.function.BiConsumer;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
public class Zipped implements Iterable.ZippedEntry> {
private final Iterator left;
private final Iterator right;
private Zipped(Iterator left, Iterator right) {
this.left = left;
this.right = right;
}
public static Zipped zip(Iterable left, Iterable right) {
return new Zipped<>(left.iterator(), right.iterator());
}
public static Zipped zip(Stream left, Iterable right) {
return new Zipped<>(left.iterator(), right.iterator());
}
public static Zipped zip(Stream left, Stream right) {
return new Zipped<>(left.iterator(), right.iterator());
}
public static Zipped zip(Iterable left, Stream right) {
return new Zipped<>(left.iterator(), right.iterator());
}
private int index = 0;
@Override
public Iterator iterator() {
return new Iterator() {
@Override
public boolean hasNext() {
return hasLeft() && hasRight();
}
@Override
public ZippedEntry next() {
return new ZippedEntry(index++, left.next(), right.next());
}
};
}
public Zipped forEachElement(BiConsumer consumer) {
forEach(e -> consumer.accept(e.left(), e.right()));
return this;
}
public boolean hasLeft() {
return left.hasNext();
}
public boolean hasRight() {
return right.hasNext();
}
public Iterable left() {
return () -> left;
}
public Iterable right() {
return () -> right;
}
public Zipped forEachElementWithIndex(IndexedBiConsumer consumer) {
forEach(e -> consumer.accept(e.index(), e.left(), e.right()));
return this;
}
public Stream stream() {
return StreamSupport.stream(spliterator(), false);
}
public int index() {
return index;
}
@FunctionalInterface
public interface IndexedBiConsumer {
void accept(int index, L left, R right);
}
public class ZippedEntry {
private final int index;
private final L left;
private final R right;
public ZippedEntry(int index, L left, R right) {
this.left = left;
this.right = right;
this.index = index;
}
public int index() {
return index;
}
public L left() {
return left;
}
public R right() {
return right;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy