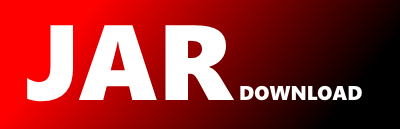
com.github.leeonky.map.BaseConverter Maven / Gradle / Ivy
package com.github.leeonky.map;
import com.github.leeonky.util.BeanClass;
import ma.glasnost.orika.CustomConverter;
import java.util.*;
import java.util.stream.Collectors;
abstract class BaseConverter extends CustomConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy