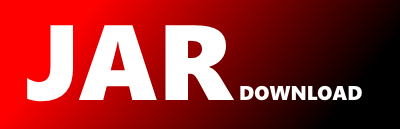
com.github.lemfi.kest.rabbitmq.builder.RabbitMQMessageExecutionBuilder.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of step-rabbitmq Show documentation
Show all versions of step-rabbitmq Show documentation
Backends testing with Kotlin
@file:Suppress("FunctionName", "unused")
package com.github.lemfi.kest.rabbitmq.builder
import com.github.lemfi.kest.core.builder.ExecutionBuilder
import com.github.lemfi.kest.core.model.Execution
import com.github.lemfi.kest.rabbitmq.executor.RabbitMQMessageExecution
import com.github.lemfi.kest.rabbitmq.model.RabbitMQPublicationProperties
import com.github.lemfi.kest.rabbitmq.model.rabbitMQProperty
import java.util.Date
class RabbitMQMessageExecutionBuilder : ExecutionBuilder {
fun publish(message: () -> String) = RabbitMQMessage(message()).also { this.message = it }
infix fun RabbitMQMessage.`to exchange`(exchange: String) = also { it.exchange = exchange }
infix fun RabbitMQMessage.`with routing key`(routingKey: String?) = also { it.routingKey = routingKey }
infix fun RabbitMQMessage.`with headers`(headers: Map) = also { it.headers = headers }
infix fun RabbitMQMessage.`with properties`(propertiesBuilder: RabbitMQPropertiesBuilder.() -> Unit) =
also { it.properties = RabbitMQPropertiesBuilder().apply(propertiesBuilder).build() }
private lateinit var message: RabbitMQMessage
@Suppress("MemberVisibilityCanBePrivate")
var connection = rabbitMQProperty { connection }
@Suppress("MemberVisibilityCanBePrivate")
var vhost = rabbitMQProperty { vhost }
override fun toExecution(): Execution {
return RabbitMQMessageExecution(
message.message,
connection,
vhost,
message.exchange ?: rabbitMQProperty { exchange },
requireNotNull(message.routingKey) { "please give a routing key for publishing a message" },
message.headers,
message.properties,
)
}
}
data class RabbitMQMessage(
val message: String,
var routingKey: String? = null,
var exchange: String? = null,
var headers: Map = mapOf(),
var properties: RabbitMQPublicationProperties? = null,
)
class RabbitMQPropertiesBuilder {
var contentType: String? = null
var contentEncoding: String? = null
var deliveryMode: Int? = null
var priority: Int? = null
var correlationId: String? = null
var replyTo: String? = null
var type: String? = null
var messageId: String? = null
var expiration: String? = null
var timestamp: Date? = null
var userId: String? = null
var appId: String? = null
fun build() =
RabbitMQPublicationProperties(
deliveryMode = deliveryMode,
type = type,
contentType = contentType,
contentEncoding = contentEncoding,
messageId = messageId,
correlationId = correlationId,
replyTo = replyTo,
expiration = expiration,
timestamp = timestamp,
userId = userId,
appId = appId
)
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy