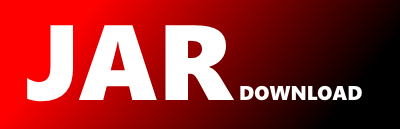
com.github.lespaul361.commons.commonroutines.boxbuilding.BoxUtilities Maven / Gradle / Ivy
/*
* Copyright (C) 2019 Charles Hamilton
*
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with this program. If not, see .
*/
package com.github.lespaul361.commons.commonroutines.boxbuilding;
import java.awt.Component;
import java.awt.Dimension;
import java.util.Arrays;
import java.util.List;
import javax.swing.Box;
import javax.swing.JButton;
import javax.swing.JLabel;
import javax.swing.JTextField;
/**
* A class with helper methods to make Boxes
*/
public class BoxUtilities {
/**
* Creates a {@link Box} with a label and a {@link JTextField}, a space
* given space (0 and less is ignored), an extra gap to align (0 and less is
* ignored), a set size for the {@link JTextField}
*
* @param lbl the {@link JLabel}
* @param sizeText the preffered size {@link Dimension} of the text box
* @param sizeMax the maximum size {@link Dimension} of the text box
* @param sizeMin the minimum size {@link Dimension} of the text box
* @param leftStrut the space from the left
* @param extra more space from the left to align this {@link Box}
* @param textField the {@link JTextField}
* @return a {@link Box}
*/
public static Box createBox(JLabel lbl, Dimension sizeText, Dimension sizeMax,
Dimension sizeMin, int leftStrut, int extra, JTextField textField) {
textField.setPreferredSize(sizeText);
textField.setMinimumSize(sizeMin);
textField.setMaximumSize(sizeMax);
Box boxH = Box.createHorizontalBox();
if (leftStrut > 0) {
boxH.add(Box.createHorizontalStrut(leftStrut));
}
if (extra > 0) {
boxH.add(Box.createHorizontalStrut(extra));
}
boxH.add(lbl);
boxH.add(textField);
if (leftStrut > 0) {
boxH.add(Box.createHorizontalStrut(leftStrut));
}
return boxH;
}
/**
* Creates a {@link Box} with a label and a {@link JButton}, a space given
* space (0 and less is ignored), a space between the {@link JLabel} and the
* {@link JButton} (0 and less is ignored), adds extra space to line it up
* (0 and less is ignored)
*
* @param lbl the {@link JLabel}
* @param leftStrut the space from the left
* @param separatorStrut the space between the {@link JLabel} and the
* {@link JButton}
* @param extra more space from the left to align this {@link Box}
* @param btn the {@link JButton}
* @return a {@link Box}
*/
public static Box createBox(JLabel lbl, int leftStrut, int separatorStrut, int extra, JButton btn) {
Box boxH = Box.createHorizontalBox();
if (leftStrut > 0) {
boxH.add(Box.createHorizontalStrut(leftStrut));
}
if (extra > 0) {
boxH.add(Box.createHorizontalStrut(extra));
}
boxH.add(lbl);
if (separatorStrut > 0) {
boxH.add(Box.createHorizontalStrut(separatorStrut));
}
boxH.add(btn);
return boxH;
}
/**
* Creates a {@link Box} with a label and a {@link JButton}, a space given
* space (0 and less is ignored), a space between the {@link JLabel} and the
* {@link JButton} (0 and less is ignored), adds extra space to line it up
* (0 and less is ignored)
*
* @param text the text to show
* @param leftStrut the space from the left
* @param separatorStrut the space between the {@link JLabel} and the
* {@link JButton}
* @param extra more space from the left to align this {@link Box}
* @param btn the {@link JButton}
* @return a {@link Box}
*/
public static Box createBox(String text, int leftStrut, int separatorStrut, int extra, JButton btn) {
return createBox(new JLabel(text), leftStrut, separatorStrut, extra, btn);
}
/**
* Sets all the {@link Dimension}s of the component to one {@link Dimension}
*
* @param c the {@link Component}
* @param sz the {@link Dimension}
*/
public static void setSize(Component c, Dimension sz) {
c.setPreferredSize(sz);
c.setMinimumSize(sz);
c.setMaximumSize(sz);
}
/**
* Sets all the {@link JButton}s to the size of the largest {@link JButton}
*
* @param buttons the {@link JButton}s
*/
public static void setLargestButtonSize(JButton... buttons) {
setLargestButtonSize(Arrays.asList(buttons));
}
/**
* Sets all the {@link JButton}s to the size of the largest {@link JButton}
*
* @param buttons the {@link JButton}s
*/
public static void setLargestButtonSize(List buttons) {
int w = 0;
int h = 0;
for (JButton b : buttons) {
w = Math.max(w, b.getPreferredSize().width);
h = Math.max(h, b.getPreferredSize().height);
}
Dimension sz = new Dimension(w, h);
buttons.forEach((b) -> {
setSize(b, sz);
});
}
/**
* Sets the sizes of the {@link Component} with the preffered size as the
* minimum size and the maximum size as the maximum integer width
*
* @param c the {@link Component}
* @param pref the preffered size
*/
public static void setSizesPrefAsMinWithMaxWidth(Component c, Dimension pref) {
Dimension max = new Dimension(Integer.MAX_VALUE, pref.height);
setSizes(c, pref, pref, max);
}
private static void setSizes(Component c, Dimension min, Dimension pref, Dimension max) {
c.setMinimumSize(min);
c.setPreferredSize(pref);
c.setMaximumSize(max);
}
/**
* Gets the largest width of the given {@link Component}s
*
* @param components the {@link Component}s
* @return an integer with the size
*/
public static int getLongestLength(Component... components) {
return getLongestLength(Arrays.asList(components));
}
/**
* Gets the largest width of the given {@link Component}s
*
* @param components the {@link Component}s
* @return an integer with the size
*/
public static int getLongestLength(List components) {
int w = 0;
for (Component c : components) {
w = Math.max(w, c.getPreferredSize().width);
}
return w;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy