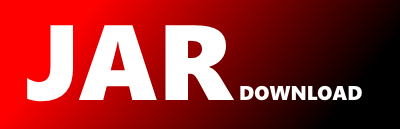
com.github.levkoposc.Tools Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jtools Show documentation
Show all versions of jtools Show documentation
JTools is simple tools for Java
package com.github.levkoposc;
import com.github.levkoposc.atomic.AtomicRun;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.concurrent.atomic.AtomicReference;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
public class Tools {
/**TOOLS*/
public static FileTools file = new FileTools();
public static SecurityTools security = new SecurityTools();
public static ReflectionTools reflection = new ReflectionTools();
/**PRIVATE*/
private static final Map threads = new HashMap<>();
public static void print(Object object) {
System.out.print(object);
}
public static void println(Object object) {
System.out.println(object);
}
public static T atomic(Supplier supplier){
AtomicReference atomic = new AtomicReference<>();
atomic.set(supplier.get());
return atomic.get();
}
public static T atomic(Supplier function, AtomicRun atomicRun){
AtomicReference atomic = new AtomicReference<>();
atomic.set(atomicRun.get(function.get()));
return atomic.get();
}
public static T atomic(Function, ?> function, AtomicRun atomicRun){
AtomicReference atomic = new AtomicReference<>();
function.apply((value)->{
atomic.set(atomicRun.get(value));
});
return atomic.get();
}
public static T[] array(T... array){
return array;
}
public static List list(T... list){
return Arrays.asList(list);
}
public static Thread getThread(String name){
return threads.get(name);
}
public static void runOnThread(String name, Runnable runnable){
Thread thread = new Thread(()->{
runnable.run();
threads.remove(name);
});
threads.put(name, thread);
thread.setName(name);
thread.start();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy