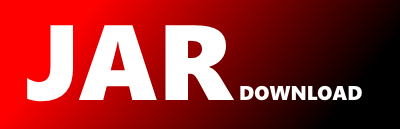
org.apache.lucene.store.jdbc.dialect.Dialect Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compass Show documentation
Show all versions of compass Show documentation
Compass Search Engine Framework
/*
* Copyright 2004-2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.lucene.store.jdbc.dialect;
import org.apache.lucene.store.jdbc.JdbcDirectorySettings;
/**
* A database specific abstraction. All other dialects must extend this Dialect.
*
* @author kimchy
*/
public abstract class Dialect {
/**
* Process settings and apply any dialect related changes. Defaults to
* do nothing.
*/
public void processSettings(JdbcDirectorySettings settings) {
}
/**
* Does the database (or the jdbc driver) supports transactional blob. Is so,
* can be used with the {@link org.apache.lucene.store.jdbc.index.FetchPerTransactionJdbcIndexInput}.
* Defaults to false
.
*/
public boolean supportTransactionalScopedBlobs() {
return false;
}
/**
* Does the database support "if exists" before the table name when constructing
* a sql drop for the table. Defaults to false.
*/
public boolean supportsIfExistsBeforeTableName() {
return false;
}
/**
* Does the database support "if exists" after the table name when constructing
* a sql drop for the table. Defaults to false
.
*/
public boolean supportsIfExistsAfterTableName() {
return false;
}
/**
* Does the dialect support a special query to check if a table exists.
* Defaults to false
.
*/
public boolean supportsTableExists() {
return false;
}
/**
* If the dialect support a special query to check if a table exists, the actual
* sql that is used to perform it. Defaults to throw an Unsupported excetion (see
* {@link #supportsTableExists()}.
*/
public String sqlTableExists(String catalog, String schemaName) {
throw new UnsupportedOperationException("Not sql provided to define if a table exists");
}
/**
* Does the database require using an InputStream
to insert a blob,
* or the setBlob
method. Defaults to true
.
*/
public boolean useInputStreamToInsertBlob() {
return true;
}
/**
* Do we need to perform a special check to see if the lock already exists in the database, or should
* we try and insert it without checking. Defaults to true
.
*/
public boolean useExistsBeforeInsertLock() {
return true;
}
/**
* The opening quote for a quoted identifier. Defaults to ".
*/
public char openQuote() {
return '"';
}
/**
* The closing quote for a quoted identifier . Defaults to ".
*/
public char closeQuote() {
return '"';
}
/**
* Some database require quoting the blob in selects
*/
public String openBlobSelectQuote() {
return "";
}
/**
* Some database require quoting the blob in selects
*/
public String closeBlobSelectQuote() {
return "";
}
/**
* Completely optional cascading drop clause
*/
public String getCascadeConstraintsString() {
return "";
}
/**
* Does the database supports select ... for update sql clause?
*/
public abstract boolean supportsForUpdate();
/**
* Does this dialect support the FOR UPDATE
syntax? Defaults to for update
.
*/
public String getForUpdateString() {
return " for update";
}
/**
* Does this dialect support the Oracle-style FOR UPDATE NOWAIT
syntax?
* Defaults to {@link #getForUpdateString()}.
*/
public String getForUpdateNowaitString() {
return getForUpdateString();
}
/**
* The type of the table that is created. Defaults to an empty string.
*/
public String getTableTypeString() {
return "";
}
/**
* Does the database supports a query for the current timestamp. Defaults to false
.
*/
public boolean supportsCurrentTimestampSelection() {
return false;
}
/**
* The database current time stamp select query.
*/
public String getCurrentTimestampSelectString() {
throw new UnsupportedOperationException("Database not known to define a current timestamp function");
}
/**
* If the current timestamp select queyr is a callable query or not.
*/
public boolean isCurrentTimestampSelectStringCallable() {
throw new UnsupportedOperationException("Database not known to define a current timestamp function");
}
/**
* The database current timestamp function that is used with several sql updates.
*/
public abstract String getCurrentTimestampFunction();
/**
* The database varchar type for the given length. The length is in chars.
*/
public abstract String getVarcharType(int length);
/**
* The database blob type for the given length. The length is in KB.
*/
public abstract String getBlobType(long length);
/**
* The database number type.
*/
public abstract String getNumberType();
/**
* The database TIMESTAMP type.
*/
public abstract String getTimestampType();
/**
* The database BIT type.
*/
public abstract String getBitType();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy