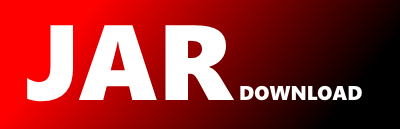
org.compass.core.util.IdentityHashSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of compass Show documentation
Show all versions of compass Show documentation
Compass Search Engine Framework
/*
* Copyright 2004-2009 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.compass.core.util;
import java.util.AbstractSet;
import java.util.IdentityHashMap;
import java.util.Iterator;
/**
* @author kimchy
*/
public class IdentityHashSet extends AbstractSet {
private transient IdentityHashMap map;
// Dummy value to associate with an Object in the backing Map
private static final Object PRESENT = new Object();
/**
* Constructs a new, empty set; the backing HashMap instance has
* default initial capacity (16) and load factor (0.75).
*/
public IdentityHashSet() {
map = new IdentityHashMap();
}
/**
* Returns an iterator over the elements in this set. The elements
* are returned in no particular order.
*
* @return an Iterator over the elements in this set.
* @see java.util.ConcurrentModificationException
*/
public Iterator iterator() {
return map.keySet().iterator();
}
/**
* Returns the number of elements in this set (its cardinality).
*
* @return the number of elements in this set (its cardinality).
*/
public int size() {
return map.size();
}
/**
* Returns true if this set contains no elements.
*
* @return true if this set contains no elements.
*/
public boolean isEmpty() {
return map.isEmpty();
}
/**
* Returns true if this set contains the specified element.
*
* @param o element whose presence in this set is to be tested.
* @return true if this set contains the specified element.
*/
public boolean contains(Object o) {
return map.containsKey(o);
}
/**
* Adds the specified element to this set if it is not already
* present.
*
* @param o element to be added to this set.
* @return true if the set did not already contain the specified
* element.
*/
public boolean add(E o) {
return map.put(o, PRESENT) == null;
}
/**
* Removes the specified element from this set if it is present.
*
* @param o object to be removed from this set, if present.
* @return true if the set contained the specified element.
*/
public boolean remove(Object o) {
return map.remove(o) == PRESENT;
}
/**
* Removes all of the elements from this set.
*/
public void clear() {
map.clear();
}
/**
* Returns a shallow copy of this HashSet instance: the elements
* themselves are not cloned.
*
* @return a shallow copy of this set.
*/
public Object clone() {
try {
IdentityHashSet newSet = (IdentityHashSet) super.clone();
newSet.map = (IdentityHashMap) map.clone();
return newSet;
} catch (CloneNotSupportedException e) {
throw new InternalError();
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy