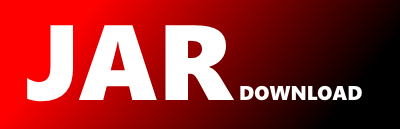
rest.RestCucumberFeatureLoader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-cucumber Show documentation
Show all versions of rest-cucumber Show documentation
Rest Cucumber allows you to attach a rest client for retrieval of Cucumber feature files and posting of Cucumber test results
package rest;
import java.io.PrintStream;
import java.util.*;
import cucumber.runtime.FeatureBuilder;
import cucumber.runtime.io.Resource;
import cucumber.runtime.io.ResourceLoader;
import cucumber.runtime.model.CucumberFeature;
import cucumber.runtime.model.PathWithLines;
public class RestCucumberFeatureLoader {
public static final String REST_CLIENT_KEY = "rest client";
private RestCucumberFeatureLoader() {
}
public static List load(ResourceLoader resourceLoader,
List featurePaths, List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy