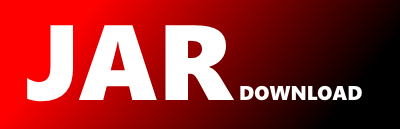
rest.Summariser Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-cucumber Show documentation
Show all versions of rest-cucumber Show documentation
Rest Cucumber allows you to attach a rest client for retrieval of Cucumber feature files and posting of Cucumber test results
package rest;
import java.util.*;
import cucumber.runtime.model.CucumberFeature;
public class Summariser {
private static final String SCENARIO = "Scenario:";
private static final String SCENARIO_INTRO = "Scenario: This is an example";
private Map results;
private Map features;
private RestRuntime runtime;
private List restScenarioList;
private List containers;
public Summariser(Map results, Map features,
RestRuntime runtime) {
this.results = results;
this.features = features;
this.runtime = runtime;
containers = new ArrayList();
runReport();
}
public List getScenarioResults() {
return restScenarioList;
}
public List getContainers() {
return containers;
}
private Map createMapOfScenarios() {
Map scenarios = new HashMap();
for (String k : features.keySet()) {
String scenario = getScenarioString(k);
scenarios.put(scenario, features.get(k));
}
return scenarios;
}
private void runReport() {
List resultList = new ArrayList();
Set r = results.keySet();
List res = new ArrayList(r);
for (int i = 0; i < res.size(); i++) {
String key = res.get(i);
String step = "[" + i + "]" + getStepString(key);
String scenario = getScenarioString(key);
String result = results.get(key);
String resultString = "[" + scenario + "],[" + step + "],[" + result + "]";
resultList.add(resultString);
}
createRestResultList(resultList);
}
private void createRestResultList(List results) {
Map scenarios = createMapOfScenarios();
restScenarioList = new ArrayList();
for (String scenario : scenarios.keySet()) {
RestScenarioResult restScenarioResult =
createRestResultForScenario(scenario, results);
restScenarioList.add(restScenarioResult);
CucumberFeature cucumberFeature = scenarios.get(scenario);
String issueKey = getIssueKeyFromCucumberFeature(cucumberFeature);
int index = checkIfContainerAlreadyExistsForIssueKey(issueKey);
if (index == -1) {
createNewContainer(cucumberFeature, restScenarioResult);
} else {
addToExistingContainer(index, restScenarioResult);
}
}
}
private String getIssueKeyFromCucumberFeature(CucumberFeature cucumberFeature) {
String issueKey = cucumberFeature.getPath();
if (!issueKey.contains(",")) {
throw new IllegalArgumentException(
"Issue key hass not been assigned to the cucumber feature correctly.");
}
issueKey = issueKey.split(",")[0];
issueKey = issueKey.replace("[", "");
return issueKey;
}
private int checkIfContainerAlreadyExistsForIssueKey(String issueKey) {
int index = -1;
for (int i = 0; i < containers.size(); i++) {
CucumberFeatureResultContainer c = containers.get(i);
if (issueKey.equals(c.getIssueKey())) {
index = i;
}
}
return index;
}
private void createNewContainer(CucumberFeature cucumberFeature,
RestScenarioResult restScenarioResult) {
CucumberFeatureResultContainer aNewContainer =
new CucumberFeatureResultContainer(cucumberFeature);
aNewContainer.addResult(restScenarioResult);
containers.add(aNewContainer);
runtime.addContainer(aNewContainer);
}
private void addToExistingContainer(int index, RestScenarioResult restScenarioResult) {
CucumberFeatureResultContainer aExistingContainer = containers.get(index);
aExistingContainer.addResult(restScenarioResult);
}
private RestScenarioResult createRestResultForScenario(String scenario,
List results) {
Map stepResultMap = new HashMap();
for (String s : results) {
String[] scenarioStepResultData = s.split(",");
String scenarioName = scenarioStepResultData[0];
scenarioName = scenarioName.replace("[", "");
scenarioName = scenarioName.replace("]", "");
if (scenarioName.equalsIgnoreCase(scenario)) {
String step = scenarioStepResultData[1];
String result = scenarioStepResultData[2];
stepResultMap.put(step, result);
}
}
return new RestScenarioResult(scenario, stepResultMap);
}
private String getStepString(String s) {
if (!s.contains(SCENARIO)) {
s = SCENARIO_INTRO + s;
}
String[] array = s.split(SCENARIO);
return array[0];
}
private String getScenarioString(String s) {
if (!s.contains(SCENARIO)) {
s = SCENARIO_INTRO + s;
}
String[] array = s.split(SCENARIO);
return SCENARIO + " " + array[1];
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy