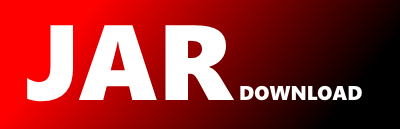
com.github.libgraviton.gdk.serialization.JsonPatcher Maven / Gradle / Ivy
package com.github.libgraviton.gdk.serialization;
import com.fasterxml.jackson.databind.JsonNode;
import com.flipkart.zjsonpatch.JsonDiff;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.util.Map;
import java.util.WeakHashMap;
/**
* Keeps track of POJOs there were deserialized via Response (with its JsonNode in its original form),
* so that the JSON Patch Diff can be calculated between the original and the current object state.
*
* @author List of contributors {@literal }
* @see http://swisscom.ch
* @version $Id: $Id
*/
public class JsonPatcher {
private JsonPatcher() {
}
private static final Logger LOG = LoggerFactory.getLogger(JsonPatcher.class);
// Since we use WeakHashMap, we don't need to worry about housekeeping.
// Whenever a map key is no longer referenced, the entry will automatically be removed from this map.
private static Map
© 2015 - 2024 Weber Informatics LLC | Privacy Policy