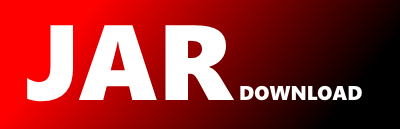
com.github.libgraviton.workerbase.Worker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of worker-base Show documentation
Show all versions of worker-base Show documentation
A base library to simplify the creation of Graviton queue workers.
/**
* connects to the queue and subscribes the WorkerConsumer on the queue
*/
package com.github.libgraviton.workerbase;
import com.fasterxml.jackson.jr.ob.JSON;
import com.fasterxml.jackson.jr.ob.impl.DeferredMap;
import com.github.libgraviton.messaging.exception.CannotConnectToQueue;
import com.github.libgraviton.messaging.exception.CannotRegisterConsumer;
import com.github.libgraviton.workerbase.exception.GravitonCommunicationException;
import com.github.libgraviton.workerbase.exception.WorkerException;
import com.github.libgraviton.workerbase.helper.PropertiesLoader;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.IOException;
import java.util.ArrayList;
import java.util.Properties;
/**
* Worker class.
*
* @author List of contributors {@literal }
* @see http://swisscom.ch
* @version $Id: $Id
*/
public class Worker {
private static final Logger LOG = LoggerFactory.getLogger(Worker.class);
/**
* properties
*/
private Properties properties;
/**
* worker
*/
private WorkerAbstract worker;
/**
* constructor
*
* @param worker worker instance
* @throws WorkerException if setup failed.
* @throws GravitonCommunicationException whenever the worker is unable to communicate with Graviton.
*/
public Worker(WorkerAbstract worker) throws WorkerException, GravitonCommunicationException {
try {
properties = PropertiesLoader.load();
} catch (IOException e) {
throw new WorkerException(e);
}
LOG.info("Starting " + properties.getProperty("application.name") + " " + properties.getProperty("application.version"));
worker.initialize(properties);
worker.onStartUp();
this.worker = worker;
}
/**
* setup worker
*
* @throws WorkerException If connecting to queue is impossible
*/
public void run() throws WorkerException {
try {
applyVcapConfig();
} catch (IOException e) {
LOG.warn("Unable to load vcap config. Skip this step.");
}
QueueManager queueManager = getQueueManager();
try {
queueManager.connect(worker);
} catch (CannotConnectToQueue | CannotRegisterConsumer e) {
throw new WorkerException("Unable to initialize worker.", e);
}
}
/**
* Let's see if we have VCAP ENV vars that we should apply to configuration
*
*/
private void applyVcapConfig() throws IOException {
String vcap = this.getVcap();
if (vcap != null) {
DeferredMap vcapConf = (DeferredMap) JSON.std.anyFrom(vcap);
if (vcapConf.containsKey("rabbitmq-3.0")) {
@SuppressWarnings("unchecked")
DeferredMap vcapCreds = ((ArrayList) vcapConf.get("rabbitmq-3.0")).get(0);
vcapCreds = (DeferredMap) vcapCreds.get("credentials");
properties.setProperty("queue.host", vcapCreds.get("host").toString());
properties.setProperty("queue.port", vcapCreds.get("port").toString());
properties.setProperty("queue.user", vcapCreds.get("user").toString());
properties.setProperty("queue.password", vcapCreds.get("password").toString());
properties.setProperty("queue.virtualhost", vcapCreds.get("virtualhost").toString());
}
}
}
/**
* Gets the properties
*
* @return properties
*/
public Properties getProperties() {
return properties;
}
/**
* Getter for vcap config
*
* @return vcap variable
*/
public String getVcap() {
return System.getenv("VCAP_SERVICES");
}
public QueueManager getQueueManager() {
return worker.getQueueManager();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy