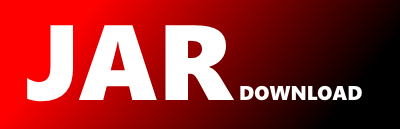
com.lowagie.text.pdf.BaseFont Maven / Gradle / Ivy
/*
* $Id: BaseFont.java 4065 2009-09-16 23:09:11Z psoares33 $
*
* Copyright 2000-2006 by Paulo Soares.
*
* The contents of this file are subject to the Mozilla Public License Version 1.1
* (the "License"); you may not use this file except in compliance with the License.
* You may obtain a copy of the License at http://www.mozilla.org/MPL/
*
* Software distributed under the License is distributed on an "AS IS" basis,
* WITHOUT WARRANTY OF ANY KIND, either express or implied. See the License
* for the specific language governing rights and limitations under the License.
*
* The Original Code is 'iText, a free JAVA-PDF library'.
*
* The Initial Developer of the Original Code is Bruno Lowagie. Portions created by
* the Initial Developer are Copyright (C) 1999, 2000, 2001, 2002 by Bruno Lowagie.
* All Rights Reserved.
* Co-Developer of the code is Paulo Soares. Portions created by the Co-Developer
* are Copyright (C) 2000, 2001, 2002 by Paulo Soares. All Rights Reserved.
*
* Contributor(s): all the names of the contributors are added in the source code
* where applicable.
*
* Alternatively, the contents of this file may be used under the terms of the
* LGPL license (the "GNU LIBRARY GENERAL PUBLIC LICENSE"), in which case the
* provisions of LGPL are applicable instead of those above. If you wish to
* allow use of your version of this file only under the terms of the LGPL
* License and not to allow others to use your version of this file under
* the MPL, indicate your decision by deleting the provisions above and
* replace them with the notice and other provisions required by the LGPL.
* If you do not delete the provisions above, a recipient may use your version
* of this file under either the MPL or the GNU LIBRARY GENERAL PUBLIC LICENSE.
*
* This library is free software; you can redistribute it and/or modify it
* under the terms of the MPL as stated above or under the terms of the GNU
* Library General Public License as published by the Free Software Foundation;
* either version 2 of the License, or any later version.
*
* This library is distributed in the hope that it will be useful, but WITHOUT
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS
* FOR A PARTICULAR PURPOSE. See the GNU Library general Public License for more
* details.
*
* If you didn't download this code from the following link, you should check if
* you aren't using an obsolete version:
* https://github.com/LibrePDF/OpenPDF
*/
package com.lowagie.text.pdf;
import com.lowagie.text.DocumentException;
import com.lowagie.text.error_messages.MessageLocalization;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.security.SecureRandom;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.StringTokenizer;
import java.util.concurrent.ConcurrentHashMap;
/**
* Base class for the several font types supported
*
* @author Paulo Soares ([email protected])
*/
public abstract class BaseFont {
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String COURIER = "Courier";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String COURIER_BOLD = "Courier-Bold";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String COURIER_OBLIQUE = "Courier-Oblique";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String COURIER_BOLDOBLIQUE = "Courier-BoldOblique";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String HELVETICA = "Helvetica";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String HELVETICA_BOLD = "Helvetica-Bold";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String HELVETICA_OBLIQUE = "Helvetica-Oblique";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String HELVETICA_BOLDOBLIQUE = "Helvetica-BoldOblique";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String SYMBOL = "Symbol";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String TIMES_ROMAN = "Times-Roman";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String TIMES_BOLD = "Times-Bold";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String TIMES_ITALIC = "Times-Italic";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String TIMES_BOLDITALIC = "Times-BoldItalic";
/**
* This is a possible value of a base 14 type 1 font
*/
public static final String ZAPFDINGBATS = "ZapfDingbats";
/**
* The maximum height above the baseline reached by glyphs in this font, excluding the height of glyphs for accented
* characters.
*/
public static final int ASCENT = 1;
/**
* The y coordinate of the top of flat capital letters, measured from the baseline.
*/
public static final int CAPHEIGHT = 2;
/**
* The maximum depth below the baseline reached by glyphs in this font. The value is a negative number.
*/
public static final int DESCENT = 3;
/**
* The angle, expressed in degrees counterclockwise from the vertical, of the dominant vertical strokes of the font.
* The value is negative for fonts that slope to the right, as almost all italic fonts do.
*/
public static final int ITALICANGLE = 4;
/**
* The lower left x glyph coordinate.
*/
public static final int BBOXLLX = 5;
/**
* The lower left y glyph coordinate.
*/
public static final int BBOXLLY = 6;
/**
* The upper right x glyph coordinate.
*/
public static final int BBOXURX = 7;
/**
* The upper right y glyph coordinate.
*/
public static final int BBOXURY = 8;
/**
* java.awt.Font property
*/
public static final int AWT_ASCENT = 9;
/**
* java.awt.Font property
*/
public static final int AWT_DESCENT = 10;
/**
* java.awt.Font property
*/
public static final int AWT_LEADING = 11;
/**
* java.awt.Font property
*/
public static final int AWT_MAXADVANCE = 12;
/**
* The underline position. Usually a negative value.
*/
public static final int UNDERLINE_POSITION = 13;
/**
* The underline thickness.
*/
public static final int UNDERLINE_THICKNESS = 14;
/**
* The strikethrough position.
*/
public static final int STRIKETHROUGH_POSITION = 15;
/**
* The strikethrough thickness.
*/
public static final int STRIKETHROUGH_THICKNESS = 16;
/**
* The recommended vertical size for subscripts for this font.
*/
public static final int SUBSCRIPT_SIZE = 17;
/**
* The recommended vertical offset from the baseline for subscripts for this font. Usually a negative value.
*/
public static final int SUBSCRIPT_OFFSET = 18;
/**
* The recommended vertical size for superscripts for this font.
*/
public static final int SUPERSCRIPT_SIZE = 19;
/**
* The recommended vertical offset from the baseline for superscripts for this font.
*/
public static final int SUPERSCRIPT_OFFSET = 20;
/**
* The font is Type 1.
*/
public static final int FONT_TYPE_T1 = 0;
/**
* The font is True Type with a standard encoding.
*/
public static final int FONT_TYPE_TT = 1;
/**
* The font is CJK.
*/
public static final int FONT_TYPE_CJK = 2;
/**
* The font is True Type with a Unicode encoding.
*/
public static final int FONT_TYPE_TTUNI = 3;
/**
* A font already inside the document.
*/
public static final int FONT_TYPE_DOCUMENT = 4;
/**
* A Type3 font.
*/
public static final int FONT_TYPE_T3 = 5;
/**
* The Unicode encoding with horizontal writing.
*/
public static final String IDENTITY_H = "Identity-H";
/**
* The Unicode encoding with vertical writing.
*/
public static final String IDENTITY_V = "Identity-V";
/**
* A possible encoding.
*/
public static final String CP1250 = "Cp1250";
/**
* A possible encoding.
*/
public static final String CP1252 = "Cp1252";
/**
* A possible encoding.
*/
public static final String CP1257 = "Cp1257";
/**
* A possible encoding.
*/
public static final String WINANSI = "Cp1252";
/**
* A possible encoding.
*/
public static final String MACROMAN = "MacRoman";
public static final int[] CHAR_RANGE_LATIN = {0, 0x17f, 0x2000, 0x206f,
0x20a0, 0x20cf, 0xfb00, 0xfb06};
public static final int[] CHAR_RANGE_ARABIC = {0, 0x7f, 0x0600, 0x067f,
0x20a0, 0x20cf, 0xfb50, 0xfbff, 0xfe70, 0xfeff};
public static final int[] CHAR_RANGE_HEBREW = {0, 0x7f, 0x0590, 0x05ff,
0x20a0, 0x20cf, 0xfb1d, 0xfb4f};
public static final int[] CHAR_RANGE_CYRILLIC = {0, 0x7f, 0x0400, 0x052f,
0x2000, 0x206f, 0x20a0, 0x20cf};
/**
* if the font has to be embedded
*/
public static final boolean EMBEDDED = true;
/**
* if the font doesn't have to be embedded
*/
public static final boolean NOT_EMBEDDED = false;
/**
* if the font has to be cached
*/
public static final boolean CACHED = true;
/**
* if the font doesn't have to be cached
*/
public static final boolean NOT_CACHED = false;
/**
* The path to the font resources.
*/
public static final String RESOURCE_PATH = "com/lowagie/text/pdf/fonts/";
/**
* The fake CID code that represents a newline.
*/
public static final char CID_NEWLINE = '\u7fff';
/**
* a not defined character in a custom PDF encoding
*/
public static final String notdef = ".notdef";
/**
* list of the 14 built in fonts.
*/
protected static final HashMap BuiltinFonts14 = new HashMap<>();
/**
* cache for the fonts already used.
*/
protected static ConcurrentHashMap fontCache = new ConcurrentHashMap<>(
500, 0.85f, 64);
static {
BuiltinFonts14.put(COURIER, PdfName.COURIER);
BuiltinFonts14.put(COURIER_BOLD, PdfName.COURIER_BOLD);
BuiltinFonts14.put(COURIER_BOLDOBLIQUE, PdfName.COURIER_BOLDOBLIQUE);
BuiltinFonts14.put(COURIER_OBLIQUE, PdfName.COURIER_OBLIQUE);
BuiltinFonts14.put(HELVETICA, PdfName.HELVETICA);
BuiltinFonts14.put(HELVETICA_BOLD, PdfName.HELVETICA_BOLD);
BuiltinFonts14
.put(HELVETICA_BOLDOBLIQUE, PdfName.HELVETICA_BOLDOBLIQUE);
BuiltinFonts14.put(HELVETICA_OBLIQUE, PdfName.HELVETICA_OBLIQUE);
BuiltinFonts14.put(SYMBOL, PdfName.SYMBOL);
BuiltinFonts14.put(TIMES_ROMAN, PdfName.TIMES_ROMAN);
BuiltinFonts14.put(TIMES_BOLD, PdfName.TIMES_BOLD);
BuiltinFonts14.put(TIMES_BOLDITALIC, PdfName.TIMES_BOLDITALIC);
BuiltinFonts14.put(TIMES_ITALIC, PdfName.TIMES_ITALIC);
BuiltinFonts14.put(ZAPFDINGBATS, PdfName.ZAPFDINGBATS);
}
protected ArrayList subsetRanges;
/**
* table of characters widths for this encoding
*/
protected int[] widths = new int[256];
/**
* encoding names
*/
protected String[] differences = new String[256];
/**
* same as differences but with the unicode codes
*/
protected char[] unicodeDifferences = new char[256];
protected int[][] charBBoxes = new int[256][];
/**
* encoding used with this font
*/
protected String encoding;
/**
* true if the font is to be embedded in the PDF
*/
protected boolean embedded;
/**
* The compression level for the font stream.
*
* @since 2.1.3
*/
protected int compressionLevel = PdfStream.DEFAULT_COMPRESSION;
/**
* true if the font must use its built in encoding. In that case the
* encoding
is only used to map a char to the position inside
* the font, not to the expected char name.
*/
protected boolean fontSpecific = true;
/**
* Forces the output of the width array. Only matters for the 14 built-in fonts.
*/
protected boolean forceWidthsOutput = false;
/**
* Converts char
directly to byte
by casting.
*/
protected boolean directTextToByte = false;
/**
* Indicates if all the glyphs and widths for that particular encoding should be included in the document.
*/
protected boolean subset = true;
protected boolean fastWinansi = false;
/**
* Custom encodings use this map to key the Unicode character to the single byte code.
*/
protected IntHashtable specialMap;
/**
* Used to build a randomized prefix for a subset name
*/
protected SecureRandom secureRandom;
/**
* Indicates if a CIDSet stream should be included in the document.
*/
protected boolean includeCidSet = true;
/**
* The font type.
*/
int fontType;
/**
* Creates new BaseFont
*/
protected BaseFont() {
}
/**
* Creates a new font. This will always be the default Helvetica font (not embedded). This method is introduced
* because Helvetica is used in many examples.
*
* @return a BaseFont object (Helvetica, Winansi, not embedded)
* @throws IOException This shouldn't occur ever
* @throws DocumentException This shouldn't occur ever
* @since 2.1.1
*/
public static BaseFont createFont() throws DocumentException, IOException {
return createFont(BaseFont.HELVETICA, BaseFont.WINANSI,
BaseFont.NOT_EMBEDDED);
}
/**
* Creates a new font. This font can be one of the 14 built in types, a Type1 font referred to by an AFM or PFM
* file, a TrueType font (simple or collection) or a CJK font from the Adobe Asian Font Pack. TrueType fonts and CJK
* fonts can have an optional style modifier appended to the name. These modifiers are: Bold, Italic and BoldItalic.
* An example would be "STSong-Light,Bold". Note that this modifiers do not work if the font is embedded. Fonts in
* TrueType collections are addressed by index such as "msgothic.ttc,1". This would get the second font (indexes
* start at 0), in this case "MS PGothic".
*
* The fonts are cached and if they already exist they are extracted from the cache, not parsed again.
*
* Besides the common encodings described by name, custom encodings can also be made. These encodings will only work
* for the single byte fonts Type1 and TrueType. The encoding string starts with a '#' followed by "simple" or
* "full". If "simple" there is a decimal for the first character position and then a list of hex values
* representing the Unicode codes that compose that encoding.
The "simple" encoding is recommended for TrueType
* fonts as the "full" encoding risks not matching the character with the right glyph if not done with care.
The
* "full" encoding is specially aimed at Type1 fonts where the glyphs have to be described by non standard names
* like the Tex math fonts. Each group of three elements compose a code position: the one byte code order in decimal
* or as 'x' (x cannot be the space), the name and the Unicode character used to access the glyph. The space must be
* assigned to character position 32 otherwise text justification will not work.
*
* Example for a "simple" encoding that includes the Unicode character space, A, B and ecyrillic:
*
*
* "# simple 32 0020 0041 0042 0454"
*
*
* Example for a "full" encoding for a Type1 Tex font:
*
*
* "# full 'A' nottriangeqlleft 0041 'B' dividemultiply 0042 32 space 0020"
*
*
* This method calls:
*
*
* createFont(name, encoding, embedded, true, null, null);
*
*
* @param name the name of the font or its location on file
* @param encoding the encoding to be applied to this font
* @param embedded true if the font is to be embedded in the PDF
* @return returns a new font. This font may come from the cache
* @throws DocumentException the font is invalid
* @throws IOException the font file could not be read
*/
public static BaseFont createFont(String name, String encoding,
boolean embedded) throws DocumentException, IOException {
return createFont(name, encoding, embedded, true, null, null, false);
}
/**
* Creates a new font. This font can be one of the 14 built in types, a Type1 font referred to by an AFM or PFM
* file, a TrueType font (simple or collection) or a CJK font from the Adobe Asian Font Pack. TrueType fonts and CJK
* fonts can have an optional style modifier appended to the name. These modifiers are: Bold, Italic and BoldItalic.
* An example would be "STSong-Light,Bold". Note that this modifiers do not work if the font is embedded. Fonts in
* TrueType collections are addressed by index such as "msgothic.ttc,1". This would get the second font (indexes
* start at 0), in this case "MS PGothic".
*
* The fonts are cached and if they already exist they are extracted from the cache, not parsed again.
*
* Besides the common encodings described by name, custom encodings can also be made. These encodings will only work
* for the single byte fonts Type1 and TrueType. The encoding string starts with a '#' followed by "simple" or
* "full". If "simple" there is a decimal for the first character position and then a list of hex values
* representing the Unicode codes that compose that encoding.
The "simple" encoding is recommended for TrueType
* fonts as the "full" encoding risks not matching the character with the right glyph if not done with care.
The
* "full" encoding is specially aimed at Type1 fonts where the glyphs have to be described by non standard names
* like the Tex math fonts. Each group of three elements compose a code position: the one byte code order in decimal
* or as 'x' (x cannot be the space), the name and the Unicode character used to access the glyph. The space must be
* assigned to character position 32 otherwise text justification will not work.
*
* Example for a "simple" encoding that includes the Unicode character space, A, B and ecyrillic:
*
*
* "# simple 32 0020 0041 0042 0454"
*
*
* Example for a "full" encoding for a Type1 Tex font:
*
*
* "# full 'A' nottriangeqlleft 0041 'B' dividemultiply 0042 32 space 0020"
*
*
* This method calls:
*
*
* createFont(name, encoding, embedded, true, null, null);
*
*
* @param name the name of the font or its location on file
* @param encoding the encoding to be applied to this font
* @param embedded true if the font is to be embedded in the PDF
* @param forceRead in some cases (TrueTypeFont, Type1Font), the full font file will be read and kept in memory if
* forceRead is true
* @return returns a new font. This font may come from the cache
* @throws DocumentException the font is invalid
* @throws IOException the font file could not be read
* @since 2.1.5
*/
public static BaseFont createFont(String name, String encoding,
boolean embedded, boolean forceRead) throws DocumentException,
IOException {
return createFont(name, encoding, embedded, true, null, null, forceRead);
}
/**
* Creates a new font. This font can be one of the 14 built in types, a Type1 font referred to by an AFM or PFM
* file, a TrueType font (simple or collection) or a CJK font from the Adobe Asian Font Pack. TrueType fonts and CJK
* fonts can have an optional style modifier appended to the name. These modifiers are: Bold, Italic and BoldItalic.
* An example would be "STSong-Light,Bold". Note that this modifiers do not work if the font is embedded. Fonts in
* TrueType collections are addressed by index such as "msgothic.ttc,1". This would get the second font (indexes
* start at 0), in this case "MS PGothic".
*
* The fonts may or may not be cached depending on the flag
* cached
. If the byte
arrays are present the font
* will be read from them instead of the name. A name is still required to identify the font type.
*
* Besides the common encodings described by name, custom encodings can also be made. These encodings will only work
* for the single byte fonts Type1 and TrueType. The encoding string starts with a '#' followed by "simple" or
* "full". If "simple" there is a decimal for the first character position and then a list of hex values
* representing the Unicode codes that compose that encoding.
The "simple" encoding is recommended for TrueType
* fonts as the "full" encoding risks not matching the character with the right glyph if not done with care.
The
* "full" encoding is specially aimed at Type1 fonts where the glyphs have to be described by non standard names
* like the Tex math fonts. Each group of three elements compose a code position: the one byte code order in decimal
* or as 'x' (x cannot be the space), the name and the Unicode character used to access the glyph. The space must be
* assigned to character position 32 otherwise text justification will not work.
*
* Example for a "simple" encoding that includes the Unicode character space, A, B and ecyrillic:
*
*
* "# simple 32 0020 0041 0042 0454"
*
*
* Example for a "full" encoding for a Type1 Tex font:
*
*
* "# full 'A' nottriangeqlleft 0041 'B' dividemultiply 0042 32 space 0020"
*
*
* @param name the name of the font or its location on file
* @param encoding the encoding to be applied to this font
* @param embedded true if the font is to be embedded in the PDF
* @param cached true if the font comes from the cache or is added to the cache if new, false if the font is
* always created new
* @param ttfAfm the true type font or the afm in a byte array
* @param pfb the pfb in a byte array
* @return returns a new font. This font may come from the cache but only if cached is true, otherwise it will
* always be created new
* @throws DocumentException the font is invalid
* @throws IOException the font file could not be read
* @since iText 0.80
*/
public static BaseFont createFont(String name, String encoding,
boolean embedded, boolean cached, byte[] ttfAfm, byte[] pfb)
throws DocumentException, IOException {
return createFont(name, encoding, embedded, cached, ttfAfm, pfb, false);
}
/**
* Creates a new font. This font can be one of the 14 built in types, a Type1 font referred to by an AFM or PFM
* file, a TrueType font (simple or collection) or a CJK font from the Adobe Asian Font Pack. TrueType fonts and CJK
* fonts can have an optional style modifier appended to the name. These modifiers are: Bold, Italic and BoldItalic.
* An example would be "STSong-Light,Bold". Note that this modifiers do not work if the font is embedded. Fonts in
* TrueType collections are addressed by index such as "msgothic.ttc,1". This would get the second font (indexes
* start at 0), in this case "MS PGothic".
*
* The fonts may or may not be cached depending on the flag
* cached
. If the byte
arrays are present the font
* will be read from them instead of the name. A name is still required to identify the font type.
*
* Besides the common encodings described by name, custom encodings can also be made. These encodings will only work
* for the single byte fonts Type1 and TrueType. The encoding string starts with a '#' followed by "simple" or
* "full". If "simple" there is a decimal for the first character position and then a list of hex values
* representing the Unicode codes that compose that encoding.
The "simple" encoding is recommended for TrueType
* fonts as the "full" encoding risks not matching the character with the right glyph if not done with care.
The
* "full" encoding is specially aimed at Type1 fonts where the glyphs have to be described by non standard names
* like the Tex math fonts. Each group of three elements compose a code position: the one byte code order in decimal
* or as 'x' (x cannot be the space), the name and the Unicode character used to access the glyph. The space must be
* assigned to character position 32 otherwise text justification will not work.
*
* Example for a "simple" encoding that includes the Unicode character space, A, B and ecyrillic:
*
*
* "# simple 32 0020 0041 0042 0454"
*
*
* Example for a "full" encoding for a Type1 Tex font:
*
*
* "# full 'A' nottriangeqlleft 0041 'B' dividemultiply 0042 32 space 0020"
*
*
* @param name the name of the font or its location on file
* @param encoding the encoding to be applied to this font
* @param embedded true if the font is to be embedded in the PDF
* @param cached true if the font comes from the cache or is added to the cache if new, false if the font is
* always created new
* @param ttfAfm the true type font or the afm in a byte array
* @param pfb the pfb in a byte array
* @param noThrow if true will not throw an exception if the font is not recognized and will return null, if false
* will throw an exception if the font is not recognized. Note that even if true an exception may be
* thrown in some circumstances. This parameter is useful for FontFactory that may have to check
* many invalid font names before finding the right one
* @return returns a new font. This font may come from the cache but only if cached is true, otherwise it will
* always be created new
* @throws DocumentException the font is invalid
* @throws IOException the font file could not be read
* @since 2.0.3
*/
public static BaseFont createFont(String name, String encoding,
boolean embedded, boolean cached, byte[] ttfAfm, byte[] pfb,
boolean noThrow) throws DocumentException, IOException {
return createFont(name, encoding, embedded, cached, ttfAfm, pfb, false,
false);
}
/**
* Creates a new font. This font can be one of the 14 built in types, a Type1 font referred to by an AFM or PFM
* file, a TrueType font (simple or collection) or a CJK font from the Adobe Asian Font Pack. TrueType fonts and CJK
* fonts can have an optional style modifier appended to the name. These modifiers are: Bold, Italic and BoldItalic.
* An example would be "STSong-Light,Bold". Note that this modifiers do not work if the font is embedded. Fonts in
* TrueType collections are addressed by index such as "msgothic.ttc,1". This would get the second font (indexes
* start at 0), in this case "MS PGothic".
*
* The fonts may or may not be cached depending on the flag
* cached
. If the byte
arrays are present the font
* will be read from them instead of the name. A name is still required to identify the font type.
*
* Besides the common encodings described by name, custom encodings can also be made. These encodings will only work
* for the single byte fonts Type1 and TrueType. The encoding string starts with a '#' followed by "simple" or
* "full". If "simple" there is a decimal for the first character position and then a list of hex values
* representing the Unicode codes that compose that encoding.
The "simple" encoding is recommended for TrueType
* fonts as the "full" encoding risks not matching the character with the right glyph if not done with care.
The
* "full" encoding is specially aimed at Type1 fonts where the glyphs have to be described by non standard names
* like the Tex math fonts. Each group of three elements compose a code position: the one byte code order in decimal
* or as 'x' (x cannot be the space), the name and the Unicode character used to access the glyph. The space must be
* assigned to character position 32 otherwise text justification will not work.
*
* Example for a "simple" encoding that includes the Unicode character space, A, B and ecyrillic:
*
*
* "# simple 32 0020 0041 0042 0454"
*
*
* Example for a "full" encoding for a Type1 Tex font:
*
*
* "# full 'A' nottriangeqlleft 0041 'B' dividemultiply 0042 32 space 0020"
*
*
* @param name the name of the font or its location on file
* @param encoding the encoding to be applied to this font
* @param embedded true if the font is to be embedded in the PDF
* @param cached true if the font comes from the cache or is added to the cache if new, false if the font is
* always created new
* @param ttfAfm the true type font or the afm in a byte array
* @param pfb the pfb in a byte array
* @param noThrow if true will not throw an exception if the font is not recognized and will return null, if false
* will throw an exception if the font is not recognized. Note that even if true an exception may
* be thrown in some circumstances. This parameter is useful for FontFactory that may have to check
* many invalid font names before finding the right one
* @param forceRead in some cases (TrueTypeFont, Type1Font), the full font file will be read and kept in memory if
* forceRead is true
* @return returns a new font. This font may come from the cache but only if cached is true, otherwise it will
* always be created new
* @throws DocumentException the font is invalid
* @throws IOException the font file could not be read
* @since 2.1.5
*/
public static BaseFont createFont(String name, String encoding,
boolean embedded, boolean cached, byte[] ttfAfm, byte[] pfb,
boolean noThrow, boolean forceRead) throws DocumentException,
IOException {
String nameBase = getBaseName(name);
encoding = normalizeEncoding(encoding);
boolean isBuiltinFonts14 = BuiltinFonts14.containsKey(name);
boolean isCJKFont = !isBuiltinFonts14 && CJKFont.isCJKFont(
nameBase, encoding);
if (isBuiltinFonts14 || isCJKFont) {
embedded = false;
} else if (encoding.equals(IDENTITY_H) || encoding.equals(IDENTITY_V)) {
embedded = true;
}
BaseFont fontFound = null;
BaseFont fontBuilt = null;
String key = name + "\n" + encoding + "\n" + embedded;
if (cached) {
fontFound = fontCache.get(key);
if (fontFound != null) {
return fontFound;
}
}
if (isBuiltinFonts14 || name.toLowerCase().endsWith(".afm")
|| name.toLowerCase().endsWith(".pfm")) {
fontBuilt = new Type1Font(name, encoding, embedded, ttfAfm, pfb,
forceRead);
fontBuilt.fastWinansi = encoding.equals(CP1252);
} else if (nameBase.toLowerCase().endsWith(".ttf")
|| nameBase.toLowerCase().endsWith(".otf")
|| nameBase.toLowerCase().indexOf(".ttc,") > 0) {
if (encoding.equals(IDENTITY_H) || encoding.equals(IDENTITY_V)) {
fontBuilt = new TrueTypeFontUnicode(name, encoding, embedded,
ttfAfm, forceRead);
LayoutProcessor.loadFont(fontBuilt, name);
} else {
fontBuilt = new TrueTypeFont(name, encoding, embedded, ttfAfm,
false, forceRead);
fontBuilt.fastWinansi = encoding.equals(CP1252);
}
} else if (isCJKFont) {
fontBuilt = new CJKFont(name, encoding, embedded);
} else if (noThrow) {
return null;
} else {
throw new DocumentException(MessageLocalization.getComposedMessage(
"font.1.with.2.is.not.recognized", name, encoding));
}
if (cached) {
fontCache.putIfAbsent(key, fontBuilt);
return fontCache.get(key);
}
return fontBuilt;
}
/**
* Creates a font based on an existing document font. The created font font may not behave as expected, depending on
* the encoding or subset.
*
* @param fontRef the reference to the document font
* @return the font
*/
public static BaseFont createFont(PRIndirectReference fontRef) {
return new DocumentFont(fontRef);
}
/**
* Gets the name without the modifiers Bold, Italic or BoldItalic.
*
* @param name the full name of the font
* @return the name without the modifiers Bold, Italic or BoldItalic
*/
protected static String getBaseName(String name) {
if (name.endsWith(",Bold")) {
return name.substring(0, name.length() - 5);
} else if (name.endsWith(",Italic")) {
return name.substring(0, name.length() - 7);
} else if (name.endsWith(",BoldItalic")) {
return name.substring(0, name.length() - 11);
} else {
return name;
}
}
/**
* Normalize the encoding names. "winansi" is changed to "Cp1252" and "macroman" is changed to "MacRoman".
*
* @param enc the encoding to be normalized
* @return the normalized encoding
*/
protected static String normalizeEncoding(String enc) {
if (enc.equals("winansi") || enc.equals("")) {
return CP1252;
} else if (enc.equals("macroman")) {
return MACROMAN;
} else {
return enc;
}
}
/**
* Gets the full name of the font. If it is a True Type font each array element will have {Platform ID, Platform
* Encoding ID, Language ID, font name}. The interpretation of this values can be found in the Open Type
* specification, chapter 2, in the 'name' table.
For the other fonts the array has a single element with {"",
* "", "", font name}.
*
* @param name the name of the font
* @param encoding the encoding of the font
* @param ttfAfm the true type font or the afm in a byte array
* @return the full name of the font
* @throws DocumentException on error
* @throws IOException on error
*/
public static String[][] getFullFontName(String name, String encoding,
byte[] ttfAfm) throws DocumentException, IOException {
String nameBase = getBaseName(name);
BaseFont fontBuilt = null;
if (nameBase.toLowerCase().endsWith(".ttf")
|| nameBase.toLowerCase().endsWith(".otf")
|| nameBase.toLowerCase().indexOf(".ttc,") > 0) {
fontBuilt = new TrueTypeFont(name, CP1252, false, ttfAfm, true,
false);
} else {
fontBuilt = createFont(name, encoding, false, false, ttfAfm, null);
}
return fontBuilt.getFullFontName();
}
/**
* Gets all the names from the font. Only the required tables are read.
*
* @param name the name of the font
* @param encoding the encoding of the font
* @param ttfAfm the true type font or the afm in a byte array
* @return an array of Object[] built with {getPostscriptFontName(), getFamilyFontName(), getFullFontName()}
* @throws DocumentException on error
* @throws IOException on error
*/
public static Object[] getAllFontNames(String name, String encoding,
byte[] ttfAfm) throws DocumentException, IOException {
String nameBase = getBaseName(name);
BaseFont fontBuilt = null;
if (nameBase.toLowerCase().endsWith(".ttf")
|| nameBase.toLowerCase().endsWith(".otf")
|| nameBase.toLowerCase().indexOf(".ttc,") > 0) {
fontBuilt = new TrueTypeFont(name, CP1252, false, ttfAfm, true,
false);
} else {
fontBuilt = createFont(name, encoding, false, false, ttfAfm, null);
}
return new Object[]{fontBuilt.getPostscriptFontName(),
fontBuilt.getFamilyFontName(), fontBuilt.getFullFontName()};
}
/**
* Gets all the entries of the namestable from the font. Only the required tables are read.
*
* @param name the name of the font
* @param encoding the encoding of the font
* @param ttfAfm the true type font or the afm in a byte array
* @return an array of Object[] built with {getPostscriptFontName(), getFamilyFontName(), getFullFontName()}
* @throws DocumentException on error
* @throws IOException on error
* @since 2.0.8
*/
public static String[][] getAllNameEntries(String name, String encoding,
byte[] ttfAfm) throws DocumentException, IOException {
String nameBase = getBaseName(name);
BaseFont fontBuilt = null;
if (nameBase.toLowerCase().endsWith(".ttf")
|| nameBase.toLowerCase().endsWith(".otf")
|| nameBase.toLowerCase().indexOf(".ttc,") > 0) {
fontBuilt = new TrueTypeFont(name, CP1252, false, ttfAfm, true,
false);
} else {
fontBuilt = createFont(name, encoding, false, false, ttfAfm, null);
}
return fontBuilt.getAllNameEntries();
}
/**
* Enumerates the postscript font names present inside a True Type Collection.
*
* @param ttcFile the file name of the font
* @return the postscript font names
* @throws DocumentException on error
* @throws IOException on error
*/
public static String[] enumerateTTCNames(String ttcFile)
throws DocumentException, IOException {
return new EnumerateTTC(ttcFile).getNames();
}
/**
* Enumerates the postscript font names present inside a True Type Collection.
*
* @param ttcArray the font as a byte
array
* @return the postscript font names
* @throws DocumentException on error
* @throws IOException on error
*/
public static String[] enumerateTTCNames(byte[] ttcArray)
throws DocumentException, IOException {
return new EnumerateTTC(ttcArray).getNames();
}
/**
* Gets the font resources.
*
* @param key the full name of the resource
* @return the InputStream
to get the resource or
* null
if not found
*/
public static InputStream getResourceStream(String key) {
return getResourceStream(key, null);
}
/**
* Gets the font resources.
*
* @param key the full name of the resource
* @param loader the ClassLoader to load the resource or null to try the ones available
* @return the InputStream
to get the resource or
* null
if not found
*/
public static InputStream getResourceStream(String key, ClassLoader loader) {
if (key.startsWith("/")) {
key = key.substring(1);
}
InputStream is = null;
if (loader != null) {
is = loader.getResourceAsStream(key);
if (is != null) {
return new BufferedInputStream(is);
}
}
// Try to use Context Class Loader to load the properties file.
try {
ClassLoader contextClassLoader = Thread.currentThread()
.getContextClassLoader();
if (contextClassLoader != null) {
is = contextClassLoader.getResourceAsStream(key);
}
} catch (Throwable e) {
}
if (is == null) {
is = BaseFont.class.getResourceAsStream("/" + key);
}
if (is == null) {
is = ClassLoader.getSystemResourceAsStream(key);
}
return is == null ? null : new BufferedInputStream(is);
}
private static void addFont(PRIndirectReference fontRef, IntHashtable hits,
ArrayList