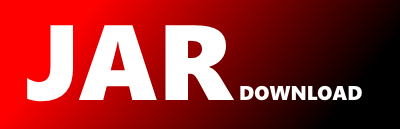
org.mybatis.generator.internal.util.HashCodeUtil Maven / Gradle / Ivy
The newest version!
package org.mybatis.generator.internal.util;
import java.lang.reflect.Array;
/**
* This class is from javapractices.com:
*
* http://www.javapractices.com/Topic28.cjp
*
* Collected methods which allow easy implementation of hashCode
.
*
* Example use case:
*
*
* public int hashCode() {
* int result = HashCodeUtil.SEED;
* //collect the contributions of various fields
* result = HashCodeUtil.hash(result, fPrimitive);
* result = HashCodeUtil.hash(result, fObject);
* result = HashCodeUtil.hash(result, fArray);
* return result;
* }
*
*/
public final class HashCodeUtil {
/**
* An initial value for a hashCode
, to which is added
* contributions from fields. Using a non-zero value decreases collisons of
* hashCode
values.
*/
public static final int SEED = 23;
/**
* booleans.
*
* @param aSeed aSeed
* @param aBoolean aBoolean
* @return hash
*/
public static int hash(int aSeed, boolean aBoolean) {
return firstTerm(aSeed) + (aBoolean ? 1 : 0);
}
/**
* chars.
* @param aSeed aSeed
* @param aChar aChar
* @return hash
*/
public static int hash(int aSeed, char aChar) {
return firstTerm(aSeed) + aChar;
}
/**
* ints.
* @param aSeed aSeed
* @param aInt aInt
* @return hash
*/
public static int hash(int aSeed, int aInt) {
/*
* Implementation Note Note that byte and short are handled by this
* method, through implicit conversion.
*/
return firstTerm(aSeed) + aInt;
}
/**
* longs.
* @param aSeed aSeed
* @param aLong aLong
* @return hash
*/
public static int hash(int aSeed, long aLong) {
return firstTerm(aSeed) + (int) (aLong ^ (aLong >>> 32));
}
/**
* floats.
* @param aSeed aSeed
* @param aFloat aFloat
* @return hash
*/
public static int hash(int aSeed, float aFloat) {
return hash(aSeed, Float.floatToIntBits(aFloat));
}
/**
* doubles.
* @param aSeed aSeed
* @param aDouble aDouble
* @return hash
*/
public static int hash(int aSeed, double aDouble) {
return hash(aSeed, Double.doubleToLongBits(aDouble));
}
/**
* aObject
is a possibly-null object field, and possibly an
* array.
*
* If aObject
is an array, then each element may be a primitive
* or a possibly-null object.
*
* @param aSeed aSeed
* @param aObject aObject
* @return hash
*/
public static int hash(int aSeed, Object aObject) {
int result = aSeed;
if (aObject == null) {
result = hash(result, 0);
} else if (!isArray(aObject)) {
result = hash(result, aObject.hashCode());
} else {
int length = Array.getLength(aObject);
for (int idx = 0; idx < length; ++idx) {
Object item = Array.get(aObject, idx);
// recursive call!
result = hash(result, item);
}
}
return result;
}
// / PRIVATE ///
private static final int fODD_PRIME_NUMBER = 37;
private static int firstTerm(int aSeed) {
return fODD_PRIME_NUMBER * aSeed;
}
private static boolean isArray(Object aObject) {
return aObject.getClass().isArray();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy