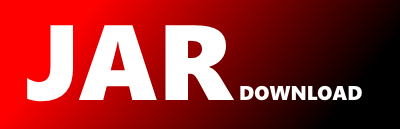
com.github.liuanxin.caches.MybatisRedisCache Maven / Gradle / Ivy
package com.github.liuanxin.caches;
import org.apache.ibatis.cache.Cache;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.springframework.data.redis.RedisConnectionFailureException;
import org.springframework.data.redis.core.RedisTemplate;
import java.util.concurrent.locks.ReadWriteLock;
import java.util.concurrent.locks.ReentrantReadWriteLock;
import java.util.regex.Pattern;
/**
*
* usr redis cache in mybatis, use RedisTemplate in spring context
*
* 1. add com.github.liuanxin.caches.RedisContextUtils in spring context
* 2. add <cache type="com.github.liuanxin.caches.MybatisRedisCache" /> in mapper.xml.
*
* @Configuration
* public class MybatisCacheConfig {
*
* @Bean
* public com.github.liuanxin.caches.RedisContextUtils setupCacheContext() {
* return new com.github.liuanxin.caches.RedisContextUtils();
* }
* }
*
*/
public class MybatisRedisCache implements Cache {
private static final Logger LOGGER = LoggerFactory.getLogger(MybatisRedisCache.class);
private static final Pattern BLANK_REGEX = Pattern.compile("\\s{2,}");
private static final String SPACE = " ";
private static RedisTemplate
© 2015 - 2024 Weber Informatics LLC | Privacy Policy