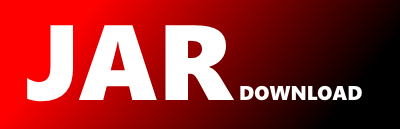
com.github.lit.support.mybatis.mapper.BaseMapper Maven / Gradle / Ivy
package com.github.lit.support.mybatis.mapper;
import com.github.lit.support.mybatis.builder.PropertyFunction;
import org.apache.ibatis.annotations.*;
import java.util.List;
/**
* @author liulu
* @version : v1.0
* date : 7/24/18 09:54
*/
public interface BaseMapper {
/**
* 新增一条记录
*
* @param entity 实体
* @return 受影响记录
*/
@InsertProvider(type = BaseSqlProvider.class, method = "insert")
@Options(useGeneratedKeys = true, keyColumn = "id")
int insert(Entity entity);
/**
* 更新一条记录
*
* @param entity entity
* @return 受影响记录
*/
@UpdateProvider(type = BaseSqlProvider.class, method = "update")
int update(Entity entity);
/**
* 删除一条记录
*
* @param id id
* @return 受影响记录
*/
@DeleteProvider(type = BaseSqlProvider.class, method = "delete")
int delete(Long id);
/**
* 根据id查询
*
* @param id id
* @return Entity
*/
@SelectProvider(type = BaseSqlProvider.class, method = "selectById")
Entity selectById(Long id);
/**
* 根据属性查询一条记录
*
* @param function property
* @param value value
* @param R
* @return Entity
*/
@SelectProvider(type = BaseSqlProvider.class, method = "selectByProperty")
Entity selectByProperty(@Param("property") PropertyFunction function, @Param("value") Object value);
/**
* 根据属性查询记录列表
*
* @param function property
* @param value value
* @param R
* @return Entity
*/
@SelectProvider(type = BaseSqlProvider.class, method = "selectByProperty")
List selectListByProperty(@Param("property") PropertyFunction function, @Param("value") Object value);
/**
* 根据查询条件查询记录
*
* @param condition condition
* @param Condition
* @return List Entity
*/
@SelectProvider(type = BaseSqlProvider.class, method = "selectByCondition")
List selectByCondition(Condition condition);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy