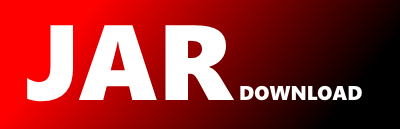
mboog.support.mapper.ReadMapper Maven / Gradle / Ivy
package mboog.support.mapper;
import mboog.support.bean.Page;
import mboog.support.example.PaginationAble;
import mboog.support.exceptions.ExampleException;
import mboog.support.exceptions.MapperException;
import java.util.Collections;
import java.util.List;
import java.util.Objects;
/**
* @param PrimaryKey
* @param Model
* @param Example
* @author LiYi
*/
public interface ReadMapper
extends BaseMapper {
long countByExample(Example example);
List selectByExample(Example example);
default Model selectByExampleSingleResult(Example example) {
List results = selectByExample(example);
int size = (results != null ? results.size() : 0);
if (size == 0) {
return null;
}
if (results.size() > 1) {
throw new MapperException("data results must single result");
}
return results.iterator().next();
}
Model selectByPrimaryKey(PrimaryKey id);
default List selectByExample(Example example, long page, long size) {
if (Objects.isNull(example)) {
throw new ExampleException("Example can't null");
}
if (example instanceof PaginationAble) {
PaginationAble temp = (PaginationAble) example;
if ("Oracle".equalsIgnoreCase(temp.getDatabaseType())) {
temp.setLimitStart((page - 1) * size);
temp.setLimitEnd(page * size);
} else {
temp.setLimitStart((page - 1) * size);
temp.setLimitEnd(size);
}
return selectByExample(example);
} else {
throw new ExampleException("Example must PaginationAble");
}
}
/**
* Pagination selectByExample
*
* @param example NOT NULL
* @param page page_no
* @param size page_size
* @return Page
*/
default Page selectPageByExample(Example example, long page, long size) {
if (Objects.isNull(example)) {
throw new ExampleException("Example can't null");
}
if (example instanceof PaginationAble) {
PaginationAble temp = (PaginationAble) example;
String orderByClause = temp.getOrderByClause();
temp.setOrderByClause(null);
long count = countByExample(example);
List list = null;
if (count > 0) {
temp.setOrderByClause(orderByClause);
list = selectByExample(example, page, size);
} else {
list = Collections.emptyList();
}
return new Page(list, count, page, size);
} else {
throw new ExampleException("Example must PaginationAble");
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy