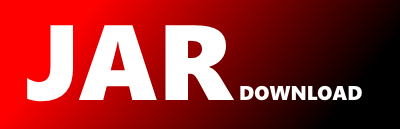
mboog.support.util.CUtil Maven / Gradle / Ivy
package mboog.support.util;
import mboog.support.example.CInterface;
import mboog.support.example.ColumnListAble;
import java.util.*;
import java.util.concurrent.ConcurrentHashMap;
/**
* C Enum util
*
* @author LiYi
*/
public abstract class CUtil {
private static final Map>, Set> C_DATA_MAP = new ConcurrentHashMap<>(128);
private static final Comparator C_COMPARATOR = Comparator.comparing(CInterface::name);
/**
* 获取分组枚举项
* @param clazz clazz
* @param types 1,2,3
* @param CInterface Set
* @return
*/
public static > Set group(Class clazz, int... types) {
E[] es = clazz.getEnumConstants();
Set set = null;
for (E e : es) {
if (e instanceof CInterface) {
CInterface c = (CInterface) e;
for (int type : types) {
if (c.getType() == type) {
if (set == null) {
set = new LinkedHashSet<>();
}
set.add(c);
break;
}
}
}
}
return set;
}
/**
* 转换Enum Item 为 CInterface
* @param clazz clazz
* @param CInterface
* @return CInterface Set
*/
public static > Set cInterfaces(Class clazz) {
E[] es = clazz.getEnumConstants();
Set set = null;
for (E e : es) {
if (e instanceof CInterface) {
CInterface c = (CInterface) e;
if (set == null) {
set = new LinkedHashSet<>();
}
set.add(c);
}
}
return set;
}
public static String joinDelimitedNames(Collection coll, boolean sort) {
if (coll == null || coll.isEmpty()) {
return "";
}
Iterator it;
// sort
if (sort) {
List list = new ArrayList<>(coll.size());
list.addAll(coll);
Collections.sort(list, C_COMPARATOR);
it = list.iterator();
} else {
it = coll.iterator();
}
// join delimited names
StringBuilder sb = new StringBuilder();
while (it.hasNext()) {
sb.append(it.next().includeColumnName());
if (it.hasNext()) {
sb.append(",");
}
}
return sb.toString();
}
public static , C extends CInterface> void includeColumns(Class clazz,
ColumnListAble columnListAble, List cs) {
columnListAble.setColumnList(null);
if (cs != null && cs.size() > 0) {
Set baseSet = new LinkedHashSet<>();
for (C c : cs) {
baseSet.add(c);
}
columnListAble.setColumnList(baseSet.isEmpty() ? null : joinDelimitedNames(baseSet, true));
}
}
public static , C extends CInterface> void includeColumns(Class clazz,
ColumnListAble columnListAble, C[] cs) {
includeColumns(clazz, columnListAble, cs == null ? new ArrayList<>() : Arrays.asList(cs));
}
public static , C extends CInterface> void excludeColumns(Class clazz,
ColumnListAble columnListAble, List cs) {
columnListAble.setColumnList(null);
if (cs != null && cs.size() > 0) {
if (!C_DATA_MAP.containsKey(clazz)) {
C_DATA_MAP.put(clazz, cInterfaces(clazz));
}
Set baseSet = new LinkedHashSet<>();
baseSet.addAll(C_DATA_MAP.get(clazz));
for (C c : cs) {
baseSet.remove(c);
}
columnListAble.setColumnList(baseSet.isEmpty() ? null : joinDelimitedNames(baseSet, true));
}
}
public static , C extends CInterface> void excludeColumns(Class clazz,
ColumnListAble columnListAble, C[] cs) {
excludeColumns(clazz, columnListAble, cs == null ? new ArrayList<>() : Arrays.asList(cs));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy