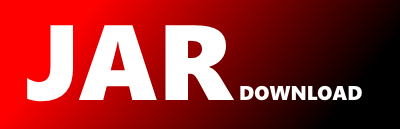
com.github.loicoudot.java4cpp.Utils Maven / Gradle / Ivy
package com.github.loicoudot.java4cpp;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.HashSet;
public final class Utils {
/**
* Creates a mutable, empty {@code ArrayList} instance.
*
* @return a new, empty {@code ArrayList}
*/
public static ArrayList newArrayList() {
return new ArrayList();
}
/**
* Creates a mutable, empty {@code HashMap} instance.
*
* @return a new, empty {@code HashMap}
*/
public static HashMap newHashMap() {
return new HashMap();
}
/**
* Creates a mutable, {@code HashMap} instance with one entry.
*
* @return a new {@code HashMap}
*/
public static HashMap newHashMap(K key, V value) {
HashMap map = new HashMap();
map.put(key, value);
return map;
}
/**
* Creates a mutable, empty {@code HashSet} instance.
*
* @return a new, empty {@code HashSet}
*/
public static HashSet newHashSet() {
return new HashSet();
}
/**
* Returns {@code true} if the given string is null or is the empty string.
*
* @param string
* a string reference to check
* @return {@code true} if the string is null or is the empty string
*/
public static boolean isNullOrEmpty(String string) {
return string == null || string.length() == 0;
}
/**
* Looks for a file in the file system path named {@code name}, if none
* exist looks for a resource named {@code name} inside the current class
* path.
*
* @param name
* a file or a resource name to find
* @return the corresponding {@code InputStream}
* @throws IOException
*/
public static InputStream getFileOrResource(String name) throws IOException {
InputStream is = null;
if (new File(name).isFile()) {
is = new FileInputStream(name);
} else {
is = Thread.currentThread().getContextClassLoader().getResourceAsStream(name);
}
if (is == null) {
throw new IOException("Failed to locate " + name);
}
return is;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy