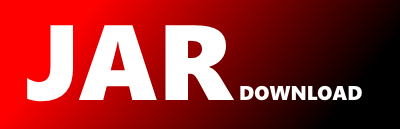
com.github.loicoudot.java4cpp.model.ClassModel Maven / Gradle / Ivy
package com.github.loicoudot.java4cpp.model;
import static com.github.loicoudot.java4cpp.Utils.newArrayList;
import static com.github.loicoudot.java4cpp.Utils.newHashSet;
import java.util.List;
import java.util.Set;
import com.github.loicoudot.java4cpp.Java4CppType;
/**
* Model that encapsulate a Java class. The model has two parts :
*
* - Type definition: contains the types mappings informations between Java
* and C++. If the class is a parametrized type,
parameters
* contains the list of parameterized type in a recursive manner. If the class
* is a generic type, parameters
contains only a
* java.lang.Object
class model. for non generic class,
* parameters
is null.
* - Class content: The instrospection results for the inner classes, enums,
* static fields, constructors and methods.
*
*
* @author Loic Oudot
*
*/
public final class ClassModel {
private ClassType type;
private List parameters;
private ClassContent content;
public ClassModel(Java4CppType type) {
this.type = new ClassType(type);
this.content = new ClassContent();
}
public void setRawClassModel(ClassModel classModel) {
type = classModel.getType();
content = classModel.getContent();
}
public ClassType getType() {
return type;
}
public List getParameters() {
return parameters;
}
public void addParameter(ClassModel parameter) {
if (parameters == null) {
parameters = newArrayList();
}
parameters.add(parameter);
}
public ClassContent getContent() {
return content;
}
public Set getIncludes() {
Set result = newHashSet();
result.addAll(getType().getIncludes());
result.addAll(getContent().getIncludes());
return result;
}
public Set getDependencies() {
Set result = newHashSet();
result.addAll(getType().getDependencies());
result.addAll(getContent().getDependencies());
return result;
}
@Override
public int hashCode() {
final int prime = 31;
int result = 1;
result = prime * result + ((type == null) ? 0 : type.getType().hashCode());
return result;
}
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null) {
return false;
}
if (getClass() != obj.getClass()) {
return false;
}
ClassModel other = (ClassModel) obj;
if (type == null) {
if (other.type != null) {
return false;
}
} else if (!type.getType().equals(other.type.getType())) {
return false;
}
return true;
}
@Override
public String toString() {
return String.format("class(%s)", type.getType());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy