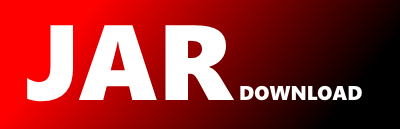
com.github.longdt.vertxorm.util.Futures Maven / Gradle / Ivy
package com.github.longdt.vertxorm.util;
import io.vertx.core.AsyncResult;
import io.vertx.core.Future;
import io.vertx.core.Handler;
import io.vertx.core.Promise;
import java.util.concurrent.CompletionException;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.LockSupport;
import java.util.function.BiConsumer;
import java.util.function.Consumer;
public final class Futures {
private Futures() {
}
public static Future toFuture(QuadConsumer>> consumer, T arg1, V arg2, U arg3) {
Promise promise = Promise.promise();
consumer.accept(arg1, arg2, arg3, promise);
return promise.future();
}
public static Future toFuture(TriConsumer>> consumer, T arg1, V arg2) {
Promise promise = Promise.promise();
consumer.accept(arg1, arg2, promise);
return promise.future();
}
public static Future toFuture(BiConsumer>> consumer, T arg) {
Promise promise = Promise.promise();
consumer.accept(arg, promise);
return promise.future();
}
public static Future toFuture(Consumer>> consumer) {
Promise promise = Promise.promise();
consumer.accept(promise);
return promise.future();
}
public static T joinNow(Future future) {
if (future.succeeded()) {
return future.result();
} else if (future.failed()) {
throw new CompletionException(future.cause());
}
return null;
}
public static T join(Future future) {
return join(future, -1, TimeUnit.NANOSECONDS);
}
public static T join(Future future, long time, TimeUnit unit) {
if (future.succeeded()) {
return future.result();
} else if (future.failed()) {
throw new CompletionException(future.cause());
}
var thread = Thread.currentThread();
future.onComplete(event -> LockSupport.unpark(thread));
if (time > 0) {
LockSupport.parkNanos(future, unit.toNanos(time));
} else {
LockSupport.park(future);
}
return joinNow(future);
}
public static R sync(QuadConsumer>> consumer, T arg1, V arg2, S arg3) {
return join(toFuture(consumer, arg1, arg2, arg3));
}
public static R sync(TriConsumer>> consumer, T arg1, V arg2) {
return join(toFuture(consumer, arg1, arg2));
}
public static R sync(BiConsumer>> consumer, T arg) {
return join(toFuture(consumer, arg));
}
public static R sync(Consumer>> consumer) {
return join(toFuture(consumer));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy