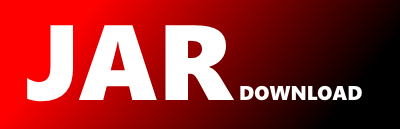
com.github.lontime.exthttp.provider.PostProvider Maven / Gradle / Ivy
package com.github.lontime.exthttp.provider;
import java.util.Map;
import com.github.lontime.base.commonj.constants.Consts;
import com.github.lontime.exthttp.common.Response;
import com.github.lontime.extjson.TypeReference;
import io.netty.handler.codec.http.DefaultHttpHeaders;
import io.netty.handler.codec.http.HttpHeaders;
import reactor.core.publisher.Mono;
/**
* PostProvider.
* @author lontime
* @since 1.0
*/
public interface PostProvider {
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param headers headers
* @param clazz clazz
* @param body body
* @return object
*/
Mono> asyncPostForObject(String name, HttpHeaders headers, String uri, Object body, TypeReference clazz);
/**
* postForObject.
* @param T
* @param uri uri
* @param reference clazz
* @param body body
* @return object
*/
default Mono> asyncPostForObject(String uri, Object body, TypeReference reference) {
return asyncPostForObject(Consts.DEFAULT_OBJECT_NAME, new DefaultHttpHeaders(), uri, body, reference);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param reference clazz
* @param body body
* @return object
*/
default Mono> asyncPostForObject(String name, String uri, Object body, TypeReference reference) {
return asyncPostForObject(name, new DefaultHttpHeaders(), uri, body, reference);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param reference clazz
* @param body body
* @param headers headers
* @return object
*/
default Mono> asyncPostForObject(String name, Map headers, String uri, Object body, TypeReference reference) {
final DefaultHttpHeaders httpHeaders = new DefaultHttpHeaders();
for (Map.Entry entry : headers.entrySet()) {
httpHeaders.add(entry.getKey(), entry.getValue());
}
return asyncPostForObject(name, httpHeaders, uri, body, reference);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param reference clazz
* @param body body
* @return object
*/
default Response postForObject(String uri, Object body, TypeReference reference) {
return postForObject(Consts.DEFAULT_OBJECT_NAME, new DefaultHttpHeaders(), uri, body, reference);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param reference clazz
* @param body body
* @return object
*/
default Response postForObject(String name, String uri, Object body, TypeReference reference) {
return postForObject(name, new DefaultHttpHeaders(), uri, body, reference);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param reference clazz
* @param body body
* @param headers headers
* @return object
*/
default Response postForObject(String name, Map headers, String uri, Object body, TypeReference reference) {
final DefaultHttpHeaders httpHeaders = new DefaultHttpHeaders();
for (Map.Entry entry : headers.entrySet()) {
httpHeaders.add(entry.getKey(), entry.getValue());
}
return postForObject(name, httpHeaders, uri, body, reference);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param headers headers
* @param reference clazz
* @param body body
* @return object
*/
default Response postForObject(String name, HttpHeaders headers, String uri, Object body, TypeReference reference) {
return asyncPostForObject(name, headers, uri, body, reference).blockOptional().orElse(null);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param headers headers
* @param clazz clazz
* @param body body
* @return object
*/
Mono> asyncPostForObject(String name, HttpHeaders headers, String uri, Object body, Class clazz);
/**
* postForObject.
* @param T
* @param uri uri
* @param clazz clazz
* @param body body
* @return object
*/
default Mono> asyncPostForObject(String uri, Object body, Class clazz) {
return asyncPostForObject(Consts.DEFAULT_OBJECT_NAME, new DefaultHttpHeaders(), uri, body, clazz);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param clazz clazz
* @param body body
* @return object
*/
default Mono> asyncPostForObject(String name, String uri, Object body, Class clazz) {
return asyncPostForObject(name, new DefaultHttpHeaders(), uri, body, clazz);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param clazz clazz
* @param body body
* @param headers headers
* @return object
*/
default Mono> asyncPostForObject(String name, Map headers, String uri, Object body, Class clazz) {
final DefaultHttpHeaders httpHeaders = new DefaultHttpHeaders();
for (Map.Entry entry : headers.entrySet()) {
httpHeaders.add(entry.getKey(), entry.getValue());
}
return asyncPostForObject(name, httpHeaders, uri, body, clazz);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param clazz clazz
* @param body body
* @return object
*/
default Response postForObject(String uri, Object body, Class clazz) {
return postForObject(Consts.DEFAULT_OBJECT_NAME, new DefaultHttpHeaders(), uri, body, clazz);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param headers headers
* @param clazz clazz
* @param body body
* @return object
*/
default Response postForObject(String uri, Map headers, Object body, Class clazz) {
return postForObject(Consts.DEFAULT_OBJECT_NAME, headers, uri, body, clazz);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param clazz clazz
* @param body body
* @return object
*/
default Response postForObject(String name, String uri, Object body, Class clazz) {
return postForObject(name, new DefaultHttpHeaders(), uri, body, clazz);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param clazz clazz
* @param body body
* @param headers headers
* @return object
*/
default Response postForObject(String name, Map headers, String uri, Object body, Class clazz) {
final DefaultHttpHeaders httpHeaders = new DefaultHttpHeaders();
for (Map.Entry entry : headers.entrySet()) {
httpHeaders.add(entry.getKey(), entry.getValue());
}
return postForObject(name, httpHeaders, uri, body, clazz);
}
/**
* postForObject.
* @param T
* @param uri uri
* @param name name
* @param headers headers
* @param clazz clazz
* @param body body
* @return object
*/
default Response postForObject(String name, HttpHeaders headers, String uri, Object body, Class clazz) {
return asyncPostForObject(name, headers, uri, body, clazz).blockOptional().orElse(null);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy